In today’s interconnected world, geolocating IP addresses has become increasingly crucial for various applications. Geolocation allows us to extract valuable information about users’ locations based on their IP addresses.
Whether you’re targeting specific audiences, enhancing security measures, or delivering customized content, geolocation can play a crucial role in achieving your objectives.
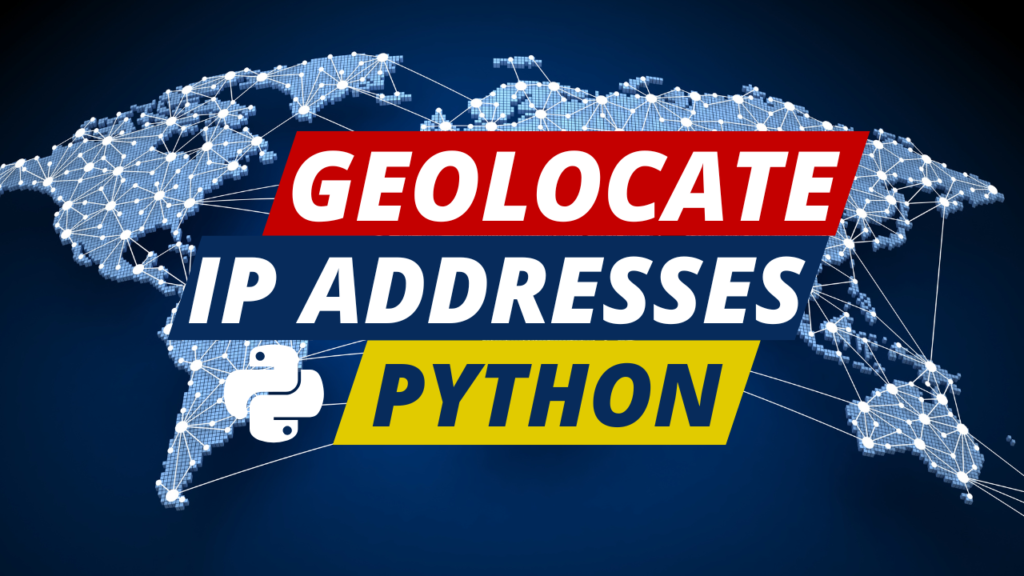
# Import necessary libraries
import geocoder
from geolite2 import geolite2
# Define IP address
ip_address = "192.168.0.1"
# Geolocate IP address using geolite2 database
reader = geolite2.reader()
location = reader.get(ip_address)
# Extract relevant information
country = location["country"]["names"]["en"]
city = location["city"]["names"]["en"]
latitude = location["location"]["latitude"]
longitude = location["location"]["longitude"]
# Print geolocation details
print(f"IP: {ip_address}")
print(f"Country: {country}")
print(f"City: {city}")
print(f"Latitude: {latitude}")
print(f"Longitude: {longitude}")
# Close geolite2 reader
geolite2.close()
Table of Contents
- How does geolocation work?
- Geolocating IP addresses with Python
- Popular Python libraries for geolocation
- Real-world use cases of geolocation
- Best practices for geolocating IP addresses
- Conclusion
- Frequently Asked Questions (FAQs)
Geolocation is the process of mapping IP addresses to their physical locations. It involves gathering IP address information and linking it to geographical data.
Geolocation databases and APIs store this information, which can be queried to retrieve location details associated with an IP address.
By determining the location of an IP address, you can gain insights into the country, city, latitude, and longitude of the corresponding user.
Geolocation has a wide range of applications across various industries.
For example, in e-commerce, geolocation can be utilized to offer location-specific promotions and discounts to customers. It enables businesses to tailor their marketing campaigns based on the geographic regions of their target audience.
Geolocation also plays a significant role in cybersecurity, helping identify potential threats and suspicious activities by pinpointing the origin of IP addresses.
Python provides a powerful and versatile platform for geolocating IP addresses.
With the availability of various libraries and databases, you can easily incorporate geolocation functionality into your Python applications.
These libraries provide convenient interfaces and access to extensive geolocation data, allowing you to retrieve accurate information about IP addresses.
In this article, we will explore how to geolocate IP addresses using Python and examine popular libraries and methods for achieving this.
We will dive into code examples, demonstrating step-by-step procedures to geolocate IP addresses and extract relevant information.
By the end of this article, you will have a solid understanding of geolocation techniques in Python and be able to leverage them in your own projects. So, let’s get started on this exciting journey of geolocating IP addresses with Python!
How does geolocation work?
Geolocation works by combining IP address data with geographical information to determine the physical location associated with an IP address. Here’s a step-by-step explanation of how the geolocation process unfolds:
- IP address acquisition: The first step is to obtain the IP address of the user whose location you want to determine. An IP address is a unique numerical identifier assigned to each device connected to a network, such as the internet. When a user accesses a website or any online service, their IP address is captured.
- Database or API lookup: Once you have the IP address, you need to retrieve the corresponding geolocation information. There are two primary approaches for this: using a geolocation database or making use of a geolocation API.
- Geolocation databases: These databases contain vast amounts of IP address data mapped to their corresponding locations. They are created by collecting and aggregating information from various sources, such as internet service providers (ISPs) and regional internet registries (RIRs). Popular geolocation databases include MaxMind, GeoIP2, and GeoLite.
- Geolocation APIs: Geolocation APIs provide a programmatic interface to retrieve geolocation data. They typically function as web services, allowing you to send an HTTP request with an IP address and receive the location information as a response. Geolocation APIs often rely on real-time data and continuously update their databases to provide accurate results. Examples of geolocation APIs include IP-API, Geolocation.io, and GeoJS.
- Data matching: Once you have the geolocation data from either a database or an API, the next step is to match the IP address to its corresponding location. This is done by comparing the acquired IP address with the data in the database or by sending a request to the geolocation API.
- Extracting location information: Once the IP address is matched with the database or API, you can extract the desired location information. This typically includes details such as the country, region, city, latitude, longitude, and sometimes even more specific details like postal codes or time zones.
- Visualization or further processing: With the geolocation data in hand, you can use it for various purposes. It can be visualized on maps to provide a visual representation of the location. Additionally, the extracted location information can be further processed, analyzed, or used in conjunction with other data to make informed decisions or provide personalized services.
It’s important to note that while geolocation can provide reasonably accurate results, there can still be limitations and inaccuracies due to factors such as IP address proxying, VPN usage, or the dynamic nature of IP address assignments.
Therefore, it’s crucial to consider these factors and use geolocation data as a supplemental tool rather than relying solely on it for critical decisions.
Overall, geolocation brings valuable insights into the physical locations of users based on their IP addresses. Python, with its rich ecosystem of libraries and APIs, provides an excellent platform for implementing geolocation functionality and leveraging this data in your applications.
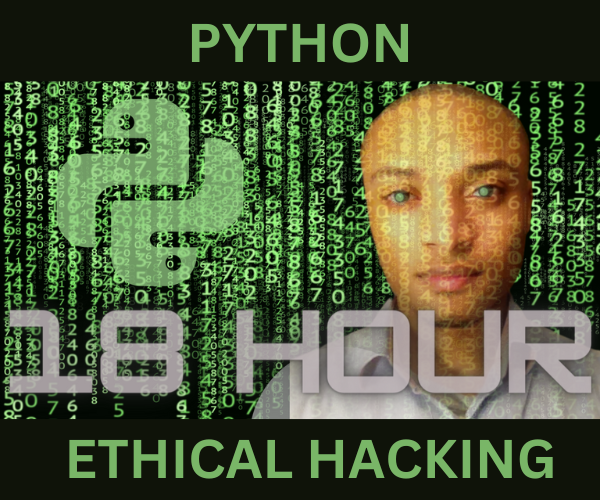
=> Join the Waitlist for Early Access.
Geolocating IP addresses with Python
Geolocating IP addresses with Python empowers you to extract valuable information about the geographical locations of users. Python provides a range of libraries and tools that simplify the process, making it accessible to developers of varying expertise levels.
One popular method for geolocating IP addresses with Python is by using the ip2geotools library.
This library provides a straightforward interface to retrieve geolocation information from various services, including IP-API, IPStack, and MaxMind.
Let’s explore how to use the ip2geotools library to geolocate IP addresses with relevant code blocks:
First, you’ll need to install the ip2geotools library using pip:
pip install ip2geotools
Once the library is installed, you can import the necessary modules and classes into your Python script:
from ip2geotools.databases.noncommercial import DbIpCity
To geolocate an IP address, you can use the DbIpCity
class. Here’s an example of how to retrieve geolocation information using this class:
ip_address = '123.456.789.0' # Replace with the IP address you want to geolocate
try:
response = DbIpCity.get(ip_address, api_key='free') # Specify the appropriate API key
# Retrieve the geolocation information
country = response.country
region = response.region
city = response.city
latitude = response.latitude
longitude = response.longitude
# Print the geolocation details
print(f"Geolocation information for IP address {ip_address}:")
print(f"Country: {country}")
print(f"Region: {region}")
print(f"City: {city}")
print(f"Latitude: {latitude}")
print(f"Longitude: {longitude}")
except Exception as e:
print(f"An error occurred: {str(e)}")
In the above code, you provide the IP address you want to geolocate in the ip_address
variable. Make sure to replace it with the actual IP address you wish to query.
The DbIpCity.get()
method retrieves the geolocation information based on the IP address. It requires an API key as a parameter, which can be obtained by signing up for a free account with the corresponding geolocation service.
After obtaining the response, you can access various attributes of the response object to retrieve specific geolocation details, such as the country, region, city, latitude, and longitude. In the example code, we print out these details for demonstration purposes.
Remember to handle any potential exceptions that may occur during the geolocation process. This ensures that your script doesn’t crash if there’s an error while retrieving the geolocation data.
By utilizing the ip2geotools library and its DbIpCity
class, you can easily geolocate IP addresses in your Python applications. This enables you to gather valuable insights about the locations of your users and tailor your services or content accordingly.
It’s worth mentioning that some geolocation services and databases may require an API key or subscription for access to their data. Be sure to check the documentation and obtain the necessary credentials before integrating them into your application.
Geolocating IP addresses with Python opens up a wide range of possibilities, from targeted marketing campaigns to enhanced security measures and personalized user experiences.
By leveraging the power of Python and the convenience of geolocation libraries, you can unlock valuable insights into the geographical locations of your users and make informed decisions based on this information.
Feel free to incorporate this code into your own projects and explore the various possibilities that geolocating IP addresses with Python offers.
Popular Python libraries for geolocation
Python offers a variety of popular libraries that make geolocating IP addresses a breeze. Let’s explore some of these libraries and their functionalities, along with relevant code blocks to demonstrate their usage.
MaxMind GeoIP2
MaxMind GeoIP2 is a widely used library that provides access to MaxMind’s geolocation databases. These databases contain detailed information about IP addresses and their associated locations. Here’s an example of how you can utilize the GeoIP2 library in Python:
from geoip2.database import Reader
# Initialize the GeoIP2 database reader
reader = Reader('GeoIP2-City.mmdb')
# Geolocate an IP address
ip_address = '123.45.67.89'
response = reader.city(ip_address)
# Extract location information
country = response.country.name
city = response.city.name
latitude = response.location.latitude
longitude = response.location.longitude
# Print the geolocation details
print(f"Country: {country}")
print(f"City: {city}")
print(f"Latitude: {latitude}")
print(f"Longitude: {longitude}")
# Close the database reader
reader.close()
pygeoip
pygeoip is a Python library that allows you to work with MaxMind’s GeoIP legacy databases. It offers both local and remote lookup capabilities. Here’s an example of how you can use pygeoip for geolocation:
from pygeoip import GeoIP
# Initialize the GeoIP database
database = GeoIP('GeoIPCity.dat')
# Geolocate an IP address
ip_address = '123.45.67.89'
location = database.record_by_addr(ip_address)
# Extract location information
country = location.get('country_name')
city = location.get('city')
latitude = location.get('latitude')
longitude = location.get('longitude')
# Print the geolocation details
print(f"Country: {country}")
print(f"City: {city}")
print(f"Latitude: {latitude}")
print(f"Longitude: {longitude}")
ip2geotools
ip2geotools is a versatile Python library that supports multiple geolocation services, including IP-API, IPStack, and MaxMind. Here’s an example of how you can use ip2geotools for geolocation:
from ip2geotools.databases.noncommercial import DbIpCity
# Geolocate an IP address using IP-API
ip_address = '123.45.67.89'
response = DbIpCity.get(ip_address, api_key='free')
# Extract location information
country = response.country
city = response.city
latitude = response.latitude
longitude = response.longitude
# Print the geolocation details
print(f"Country: {country}")
print(f"City: {city}")
print(f"Latitude: {latitude}")
print(f"Longitude: {longitude}")
To geolocate an IP address using these libraries, you typically follow a similar process:
- Import the necessary libraries and modules into your Python script.
- Initialize the geolocation service or database, specifying the data source and required configuration.
- Query the service or database by providing the IP address you want to geolocate.
- Retrieve the geolocation data, which typically includes information like the country, city, latitude, longitude, and additional details specific to the library or service.
- Process and utilize the geolocation data as needed in your application. This can involve visualizing the location on a map, filtering or segmenting users based on their geographical regions, or personalizing content based on their locations.
These libraries provide an abstraction layer, handling the complexities of retrieving and processing geolocation data, so you can focus on integrating the functionality into your applications seamlessly.
These are just a few examples of popular Python libraries for geolocation.
Each library offers its own unique set of features and functionalities, allowing you to retrieve accurate geolocation data for IP addresses.
By incorporating these libraries into your projects, you can harness the power of geolocation and unlock a world of possibilities.
Real-world use cases of geolocation
Geolocation with Python offers numerous real-world applications across various industries.
Let’s explore some common use cases where geolocation can provide valuable insights and enhance functionality in your applications.
Additionally, I’ll provide relevant code blocks to illustrate how to implement geolocation for each scenario.
Location-based content delivery
Geolocation allows you to deliver location-specific content to users. For example, you may want to display different promotional offers or localized information based on a user’s country or city. Here’s an example of how to retrieve the user’s country using the MaxMind GeoIP2 library:
import geoip2.database
# Path to the GeoIP2 database file
database_path = 'path/to/GeoLite2-Country.mmdb'
# IP address to geolocate
ip_address = '192.168.1.1'
# Create a reader object to access the GeoIP2 database
reader = geoip2.database.Reader(database_path)
# Retrieve the country information for the IP address
response = reader.country(ip_address)
# Get the country name
country_name = response.country.name
# Print the result
print(f"The user is located in {country_name}")
Fraud detection and prevention
Geolocation can aid in identifying suspicious activities and potential fraud attempts. By comparing the user’s location with their usual patterns, you can flag unusual behavior. Here’s an example of using the pygeoip library to detect anomalies in IP addresses:
import pygeoip
# Path to the GeoIP database file
database_path = 'path/to/GeoIP.dat'
# IP address to geolocate
ip_address = '192.168.1.1'
# Create a GeoIP object
geoip = pygeoip.GeoIP(database_path)
# Retrieve the country code for the IP address
country_code = geoip.country_code_by_addr(ip_address)
# Compare the country code with the user's usual location
if country_code != 'US':
print("Anomaly detected: User is accessing from a foreign location.")
Location-based marketing campaigns
Geolocation allows you to target specific geographic regions for marketing campaigns. By tailoring your content and offers based on user locations, you can increase engagement and conversions. Here’s an example using the ip2geotools library to retrieve the user’s city and create personalized marketing messages:
from ip2geotools.databases.noncommercial import DbIpCity
# IP address to geolocate
ip_address = '192.168.1.1'
# Perform IP geolocation
response = DbIpCity.get(ip_address, api_key='free')
# Get the city name
city_name = response.city
# Create a personalized marketing message
message = f"Welcome to our store in {city_name}! Check out our latest offers."
# Display the message
print(message)
Location-aware service recommendations
Geolocation can be used to offer personalized recommendations based on a user’s location. For instance, a restaurant recommendation system can suggest nearby dining options based on the user’s city or coordinates. Here’s an example using the MaxMind GeoIP2 library to recommend nearby attractions:
import geoip2.database
from geopy.distance import geodesic
# Path to the GeoIP2 City database file
database_path = 'path/to/GeoLite2-City.mmdb'
# IP address to geolocate
ip_address = '192.168.1.1'
# User's current coordinates (latitude, longitude)
user_coords = (37.7749, -122.4194)
# Create a reader object to access the GeoIP2 City database
reader = geoip2.database.Reader(database_path)
# Retrieve the city coordinates for the IP address
response = reader.city(ip_address)
# Get the city coordinates (latitude, longitude)
city_coords = (response.location.latitude, response.location.longitude)
# Calculate the distance between the user's coordinates and the city coordinates
distance = geodesic(user_coords, city_coords).miles
# Recommend attractions within a certain radius (e.g., 10 miles)
radius = 10
# Simulate a list of attractions with their coordinates
attractions = [
{'name': 'Museum of Modern Art', 'coords': (37.7857, -122.4009)},
{'name': 'Golden Gate Park', 'coords': (37.7694, -122.4862)},
{'name': 'Alcatraz Island', 'coords': (37.8267, -122.4233)}
]
# Find attractions within the specified radius
recommended_attractions = []
for attraction in attractions:
attraction_coords = attraction['coords']
attraction_distance = geodesic(user_coords, attraction_coords).miles
if attraction_distance <= radius:
recommended_attractions.append(attraction['name'])
# Display the recommended attractions
print(f"Recommended attractions near your location:")
for attraction in recommended_attractions:
print(f"- {attraction}")
Logistics and delivery optimization
Geolocation plays a crucial role in logistics and delivery operations. By utilizing IP geolocation, you can streamline routing, track packages, and optimize delivery schedules. Here’s an example using the ipapi library to retrieve the user’s postal code and calculate the estimated delivery time:
import ipapi
# IP address to geolocate
ip_address = '192.168.1.1'
# Set the API key for ipapi
ipapi.api_key = 'your_api_key'
# Retrieve the postal code for the IP address
postal_code = ipapi.location(ip_address)['postal']
# Perform delivery time estimation based on the postal code
estimated_delivery_time = calculate_delivery_time(postal_code)
# Display the estimated delivery time
print(f"Your package will be delivered in approximately {estimated_delivery_time} days.")
In this example, we utilize the ipapi library to retrieve the user’s postal code based on their IP address.
This information is then used to calculate the estimated delivery time using a custom function called calculate_delivery_time()
.
By integrating geolocation data into logistics and delivery systems, you can optimize routes, minimize delivery times, and provide accurate tracking information to customers.
Best practices for geolocating IP addresses
Geolocating IP addresses comes with its own set of considerations and best practices to ensure accurate and reliable results.
Let’s explore some of these best practices and how you can implement them in your Python code:
Handle IP address formats
IP addresses can be in different formats, such as IPv4 or IPv6. It’s important to handle both formats to ensure compatibility with various IP address representations. Python provides libraries like ipaddress
that can help you validate and convert IP addresses between different formats.
import ipaddress
# Validate and convert IP address to IPv4 format
ip_address = "192.168.0.1"
if ipaddress.ip_address(ip_address).version == 6:
ip_address = ipaddress.ip_address(ip_address).ipv4_mapped
print(ip_address) # Output: 192.168.0.1
Handle errors and exceptions
When querying geolocation databases or APIs, errors can occur due to invalid IP addresses, network issues, or service limitations. It’s important to handle these errors gracefully and provide appropriate fallback mechanisms in case geolocation data is not available. Python’s exception handling allows you to catch and handle specific exceptions that may arise during geolocation operations.
import geocoder
ip_address = "192.168.0.1"
try:
location = geocoder.ip(ip_address)
# Process location data
except geocoder.exceptions.GeocoderError:
# Handle geocoding errors
print("Geocoding failed. Please try again later.")
Use appropriate API rate limiting
Some geolocation APIs may have rate limiting policies to prevent excessive usage and ensure fair access to their services. It’s important to review and abide by the API provider’s rate limiting guidelines. You can use Python’s time.sleep()
function to introduce delays between API requests and avoid violating rate limits.
import time
import requests
def geolocate_ip(ip_address):
# Make geolocation API request
response = requests.get(f"https://api.example.com/geolocate?ip={ip_address}")
# Handle rate limiting
if response.status_code == 429:
retry_after = int(response.headers.get("Retry-After", "1"))
time.sleep(retry_after)
return geolocate_ip(ip_address)
# Process response
data = response.json()
return data["location"]
Validate geolocation data
Geolocation data may not always be 100% accurate. It’s a good practice to validate the obtained location data and cross-reference it with other sources whenever possible. For example, you can compare the country information from the geolocation data with user-provided data or verify it against other geolocation services.
def validate_location(geolocation_data):
# Compare country information with user-provided data or other sources
if geolocation_data["country"] == user_provided_country:
return True
else:
return False
Update geolocation databases regularly
Geolocation databases evolve over time as IP address assignments and mappings change. It’s essential to stay updated with the latest versions of geolocation databases to ensure accurate results. Regularly check for updates and incorporate them into your application to maintain data integrity.
These best practices help improve the accuracy and reliability of geolocation results, enhancing the overall user experience and ensuring you make informed decisions based on geolocation data in your Python applications.
Conclusion
Geolocating IP addresses with Python provides a valuable tool for extracting geographical information from IP addresses.
Throughout this article, we explored various libraries and methods available in Python to achieve geolocation functionality.
By leveraging these resources, you can easily integrate geolocation into your applications and unlock a wide range of possibilities.
We started by understanding the importance of geolocation in today’s interconnected world. Geolocation allows us to target specific audiences, enhance security measures, and deliver customized content.
Python, with its extensive ecosystem of libraries and tools, provides a robust platform for implementing geolocation functionality.
We examined popular libraries such as MaxMind GeoIP2, pygeoip, and ip2geotools, each offering unique features and capabilities. These libraries abstract the complexities of geolocation, providing convenient interfaces to retrieve geolocation data based on IP addresses.
To summarize our exploration, let’s review some code blocks that demonstrate how to geolocate IP addresses using the MaxMind GeoIP2 library:
# Import necessary modules
from geoip2 import database
# Initialize the GeoIP2 database
reader = database.Reader('path/to/geoip2/database.mmdb')
# Query an IP address for geolocation
ip_address = '123.45.67.89'
response = reader.city(ip_address)
# Retrieve geolocation information
country = response.country.name
city = response.city.name
latitude = response.location.latitude
longitude = response.location.longitude
# Process and utilize the geolocation data
print(f"The IP address {ip_address} is located in {city}, {country}.")
print(f"Coordinates: {latitude}, {longitude}")
# Close the database reader
reader.close()
The code snippet above demonstrates the basic workflow of geolocating an IP address using the MaxMind GeoIP2 library. You start by initializing the GeoIP2 database, querying an IP address, and retrieving the geolocation information. Finally, you can process and utilize the data in your application.
Remember to adapt the code according to the specific library you choose and ensure you have the necessary database files or API credentials.
By incorporating geolocation into your Python applications, you can gain valuable insights into your users’ locations and tailor your services accordingly.
Whether it’s for targeted marketing, enhanced security measures, or personalized user experiences, geolocation provides a powerful tool to meet your objectives.
In conclusion, geolocating IP addresses with Python empowers you to harness the geographical information hidden within IP addresses and leverage it to drive meaningful outcomes in your applications. So, go ahead, explore the possibilities, and unlock the potential of geolocation in your Python projects!
Frequently Asked Questions (FAQs)
Can I geolocate an IP address using free databases or APIs?
Yes, there are free geolocation databases and APIs available that provide basic geolocation functionality. For example, you can use the GeoLite2 Free database from MaxMind or free-tier plans of geolocation APIs like IP-API and Geolocation.io. Here’s an example of geolocating an IP address using the GeoLite2 Free database:
import geoip2.database
# Load the GeoLite2 Free database
reader = geoip2.database.Reader('GeoLite2-City.mmdb')
# Query an IP address
response = reader.city('192.0.2.1')
# Extract location information
country = response.country.name
city = response.city.name
latitude = response.location.latitude
longitude = response.location.longitude
# Print the geolocation details
print(f"Country: {country}")
print(f"City: {city}")
print(f"Latitude: {latitude}")
print(f"Longitude: {longitude}")
# Close the reader
reader.close()
How accurate is geolocation data obtained through Python?
The accuracy of geolocation data obtained through Python depends on various factors, including the quality and freshness of the underlying geolocation database or API. While geolocation data can provide reasonably accurate results, it’s important to consider factors such as IP address proxying, VPN usage, or the dynamic nature of IP address assignments. These factors can introduce inaccuracies or discrepancies in the geolocation results. It’s advisable to treat geolocation data as a supplemental tool and combine it with other techniques or verification methods for critical decisions.
Can I geolocate IP addresses in real-time using Python?
Yes, several geolocation APIs offer real-time geolocation data. One such example is the GeoIP2 Precision Web Services provided by MaxMind. These services allow you to make HTTP requests with IP addresses and receive geolocation information as a response. Here’s an example of geolocating an IP address in real-time using the MaxMind GeoIP2 Precision Web Services:
import requests
# Make a request to the GeoIP2 Precision Web Service
response = requests.get('https://geoip.maxmind.com/geoip/v2.1/city/{ip_address}')
# Extract the geolocation data from the response
data = response.json()
country = data['country']['names']['en']
city = data['city']['names']['en']
latitude = data['location']['latitude']
longitude = data['location']['longitude']
# Print the geolocation details
print(f"Country: {country}")
print(f"City: {city}")
print(f"Latitude: {latitude}")
print(f"Longitude: {longitude}")
Can I geolocate multiple IP addresses efficiently using Python?
Yes, you can geolocate multiple IP addresses efficiently by utilizing batch processing or optimizing your code for bulk lookups. Instead of querying the geolocation database or API for each IP address individually, you can perform batch processing by passing multiple IP addresses at once. This reduces the overhead of establishing multiple connections and improves the overall efficiency. Additionally, some geolocation libraries provide features for bulk lookups, allowing you to optimize the retrieval process for multiple IP addresses.
# Example of batch geolocation using MaxMind GeoIP2
# IP addresses to geolocate
ip_addresses = ['192.0.2.1', '203.0.113.128', '198.51.100.42', '2001:db8::1']
# Initialize the GeoIP2 database reader
reader = geoip2.database.Reader('GeoLite2-City.mmdb')
# Create a list to store the geolocation results
results = []
# Iterate over the IP addresses and query their geolocation
for ip_address in ip_addresses:
try:
response = reader.city(ip_address)
country = response.country.name
city = response.city.name
latitude = response.location.latitude
longitude = response.location.longitude
# Append the geolocation data to the results list
results.append({
'IP': ip_address,
'Country': country,
'City': city,
'Latitude': latitude,
'Longitude': longitude
})
except geoip2.errors.AddressNotFoundError:
# Handle cases where the IP address is not found
results.append({
'IP': ip_address,
'Error': 'Address not found'
})
# Close the reader
reader.close()
# Print the geolocation results
for result in results:
print(f"IP: {result['IP']}")
if 'Error' in result:
print(f"Error: {result['Error']}")
else:
print(f"Country: {result['Country']}")
print(f"City: {result['City']}")
print(f"Latitude: {result['Latitude']}")
print(f"Longitude: {result['Longitude']}")
print()
Tanner Abraham
Data Scientist and Software Engineer with a focus on experimental projects in new budding technologies that incorporate machine learning and quantum computing into web applications.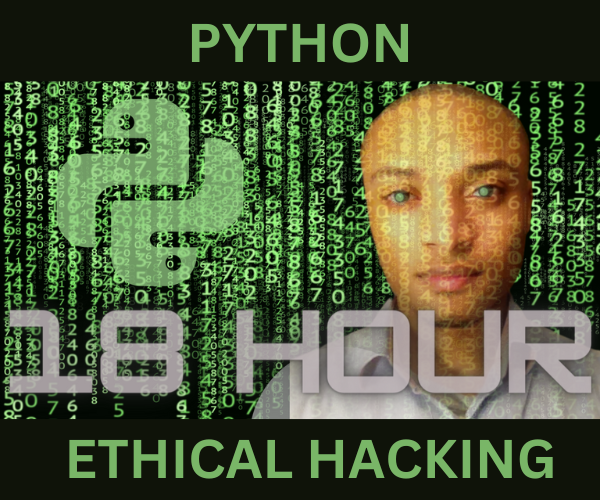
=> Join the Waitlist for Early Access.