When trading cryptocurrencies, there is one thing you should never joke about: the techniques that will make you a unique investor and, most likely, richer. You need to start trading on a recognized and authorized exchange platform for this to become a reality.
One of the best exchange sites to start trading on is Coinbase. It provides you with different options, like trading bots, to assist you in trading when you are elusive.
So what exactly is Coinbase, and what makes it one of the best exchange platforms for cryptocurrency investments?
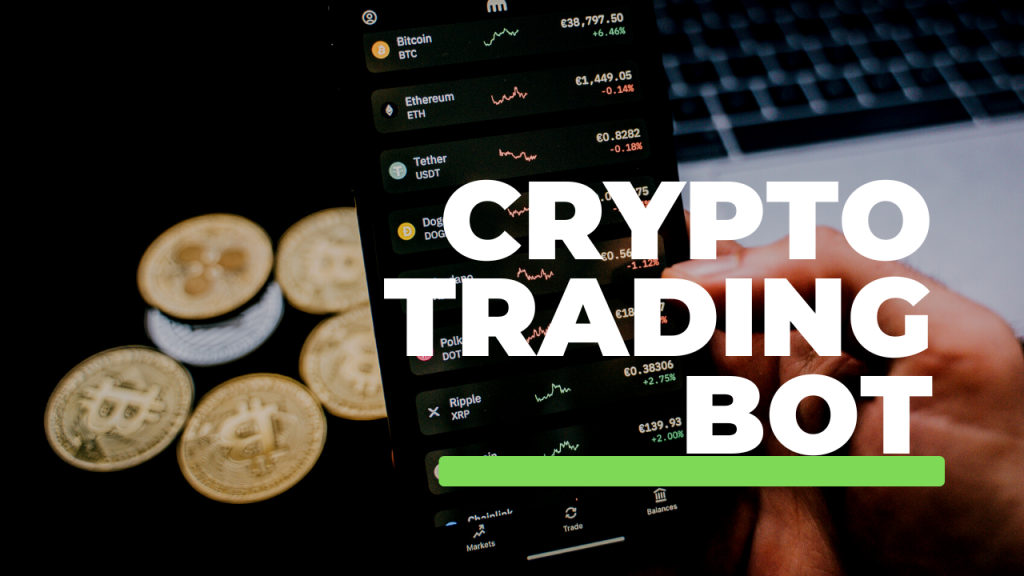
Table of Contents
- What You Should Know About the Coinbase Crypto Exchange Platform
- What are Coinbase Crypto Trading Bots?
- How Coinbase Crypto Trading Bots Works
- What are the Benefits of Coinbase Crypto Trading Bots?
- When Should You Use Crypto Trading Bots?
- Understanding the Buy Low and Sell High Strategy
- Getting Started With Coding Coinbase Crypto Trading Bots in Python
- Steps to Building Your First Coinbase Crypto Trading Bot in Python
- Sample Codes for Coinbase Crypto Trading Bots in Python
- Conclusion
What You Should Know About the Coinbase Crypto Exchange Platform
Coinbase offers a secure platform for trading and investing in cryptocurrencies. It is a huge company, with over 98 million members and $256 billion in assets on the platform.
Over 100 tradable cryptocurrencies, including Bitcoin, Ethereum, and Dogecoin, are available for customers to purchase, sell, and trade on Coinbase.
The Coinbase platform has a user-friendly layout, and any Coinbase user can access Coinbase Pro for lower costs and more functionality. It offers you more trade kinds, including limit and quit orders, unavailable on the Coinbase site, with affordable price alternatives.
Even though novices might prefer the standard Coinbase platform for straightforward buy-and-sell orders, the pro version provides expert users with additional options.
If you’re interested in cryptocurrencies, Coinbase is a tremendous choice for both experienced users and beginners. It prides itself on being an extensive cryptocurrency ecosystem with the support of over 13,000 financial institutions.
You can trade on Coinbase if you have a basic understanding of computers or if you’ve ever traded stocks using an online brokerage.
Again, Coinbase is accessible at all times because it is open twenty-four hours a day. And you can use funds from your account, a linked bank account, or a credit card to make purchases. You can access Coinbase on the web or Android and iOS mobile devices.
To make their trading experiences valuable while using the site, Coinbase gives its members a variety of options. By instructing the crypto trading bots to adhere to one of the best trading techniques, you may employ them to make your investment lucrative. For example, the buy low and sell high strategy.
What are Coinbase Crypto Trading Bots?
A piece of software called a Coinbase crypto trading bot takes over your trading position. They are automated to assist you in finding the optimum moment to buy and sell crypto. But you should always do this with a unique plan that you program the bots to follow.
By using trading bots, crypto investors can automate the purchasing and selling of positions based on important technical indicators. By using certain trading strategies, bots strive to have the highest “win rate,” or percentage of winning trades.
The fundamental goals of this program are to increase earnings while reducing risks and losses.
How Coinbase Crypto Trading Bots Works
A Coinbase cryptocurrency trading bot is programmed to follow a specific trading strategy, acting more like a human trader. They can make trades without using biased tactics since they can start and liquidate positions without jeopardizing or involving human emotions.
To own a trading bot, you must write your own code and develop a distinctive trading strategy. Many different trading techniques depend on various indicators, and if you already base your trading decisions on indicators, a trading bot might make the process more efficient.
Therefore, you can always take advantage of the services that we will discuss later in this article if you cannot code yourself.
What are the Benefits of Coinbase Crypto Trading Bots?
The cryptocurrency trading bots on Coinbase are faster than humans and optimize the utilization of each second and minute to control deals. Trading bots for cryptocurrencies would have completed trades quickly on your behalf. Other advantages are:
- Instantaneously, they carry out a significant number of computations and handle sizable transactions across numerous platforms, time zones, and markets.
- The fact that cryptocurrency trading bots follow pre-established trading rules makes them reliable. Even in the highly volatile cryptocurrency market, where you might be tempted to sell due to a dip, they maintain steady trading discipline.
- By using cryptocurrency trading bots, you may avoid the emotional ups and downs of manual trading.
- They guarantee a methodical and emotionless approach to trading.
When Should You Use Crypto Trading Bots?
Crypto trading bots are best for data collection, intelligent order routing, portfolio management, rebalancing, etc.
Again, a cryptocurrency trading bot can help you if you have trouble managing deals that are repeated by enabling you to simply copy and paste a certain task to make trades without difficulty.
It takes a lot of time and effort to do repetitive jobs; trading bots eliminate this necessity.
A trading bot analyzes the market, performs trades at the right time, and balances your portfolio every hour. It is your best option if you sometimes have a better plan but lack the time or even know when the market fits your method.
Let’s begin by implementing the buy low and sell high strategy in Python trading bots for Coinbase.
But first, let’s examine the basics of the buy low and sell high method.
Understanding the Buy Low and Sell High Strategy
The buy low, sell high investment strategy, commonly referred to as “buy the dips,” involves acquiring additional assets when the price declines and/or after it has stabilized.
The term “buying low” refers to trying to anticipate when a certain cryptocurrency will be at its lowest price and then purchasing in the belief that it will increase.
On the other hand, selling high entails determining when the market will reach its highest value and then selling the shares to benefit from it. Buying low and selling high is incredibly tough to do without technical analysis.
This approach is most advised to be employed in bear, stagnant, or bull markets when the normal trend is upward or sideways.
The key objective when purchasing dips is to purchase at a reduced price because doing so lessens the possibility that you will make expensive errors. Selling quickly and profitably is the secondary objective.
So next, we will be writing a Python code that tells a coinbase bot to buy when the price is low and sell when the price rises above a certain range.
Getting Started With Coding Coinbase Crypto Trading Bots in Python
To perform this operation, you need to have a basic knowledge of the Python programming language. Additionally, you will need a machine with intense battery life or one that is always plugged into a power source with internet access.
Python’s numerous machine-learning packages make it the perfect language for building trading bots. Python is an ideal programming language choice for both experts and beginners and offers strong libraries for financial research and visualization.
This approach works by having the trading bot evaluate a predetermined set of tickers in the portfolio before making a buy or sell decision. Price fluctuations over a given period are one possible source of data that the bot uses to arrive at this conclusion.
In particular, you must have a current version of Python installed together with a Python programming environment like Jupiter or VSC. For this procedure, you can alternatively use Google Colab.
Steps to Building Your First Coinbase Crypto Trading Bot in Python
For this process to be successful, you need to follow the following steps:
- Sign into your Coinbase account.
- Set up your API
- Connect your API and automate the trading in Python
You will need three important pieces of information from your Coinbase Pro. So to get this piece of information, follow the instructions below:
- Login into your Coinbase Pro account and click on “my account.”
- Click on API to create a new API key.
- All you need to do is copy and paste your public key, a secret key that is not to be shared with anyone, and your passphrase keys into a file in your development environment. To connect with your trading bot and receive trade commands, Coinbase employs API keys.
Once this is finished, you can install the Coinbase Pro requirements to let your Python environment make the connection.
# this will connect your bot with your Coinbase API
pip install cbpro
It’s time to begin developing in your Python coding environment once this has been installed.
Sample Codes for Coinbase Crypto Trading Bots in Python
Before you kickstart the main process, you need to ensure that you are connected to Coinbase Pro. Let’s execute some code in VSC to verify the earlier installation while also testing some of the functions this project will require.
Step 1. Testing some of the trading functions
import cbpro
# checking for products you can buy and sell
Public_client = cbpro.publicClient()
Coinbase_buy_sell = public_client.get_products()
for sb in Coinbase_buy_sell:
print(sb['id'])
# to get time
Coinbase_time = public_client.get_time()
print(Coinbase_time)
# get product order book for diffeent cryptocurrencies
Coinbase_product_order_Book = public_client.get_product_order_book('BTC-USD')
print(Coinbase_product_order_Book)
#get product ticker to see the size, trade id and so on
Coinbase_product_ticker = public_client.get_product_ticker('BTC-USD')
print(Coinbase_product_ticker)
# check the 24 hour stat
Coinbase_product_24hr_stats = public_client.get_product_ticker('BTC-USD')
print(Coinbase_product_24hr_stats)
# getting trades as generator
Coinbase_trades = public_client.get_product_trades('ETH-USD')
print(next(Coinbase_trades))
Step 2. Samples Codes to buy low and sell high in Python
Note: Ensure you store the public, private and passphrase keys as a text file in your VSC.
import cbpro
import time
# read the data from the file containing the three keys
data = open('passphrase', 'r').read().splitlines()
public = data[0]
passphrase = data[1]
private = data[2]
auth_client = cbpro.AuthenticatedClient(public, private, passphrase)
#print to check if the client is authenticated or not
print(auth_client)
print(auth_client.get_accounts())
# use this line of code to test for a single buy
# print(auth_client.buy(price="10.0", size="2.1", order_type="limit", product_id="ETH-USD"))
#print(auth_client.buy(size="2.1", order_type="market", product_id="ETH-USD"))
#print(auth_client.get_accounts())
# use this line of code to test for a single sell
#print(auth_client.sell(price="300000.00", size="10", order_type="limit", product_id="BTC-USD"))
#print(auth_client.sell(size="10", order_type="market", product_id="BTC-USD"))
sell_price = 50000
sell_amount = 0.5
buy_price = 30000
buy_amount = 0.3
while True:
cost = float(auth_client.get_product_ticker(product_id"BTC-USD")['price'])
if cost <= buy_price:
print("Order for BTC is placed as {cost:,} dipped below buying price limit of {buy_price}")
auth_client.buy(size=buy_amount, order_type="market", product_id="BTC-USD")
elif cost >= sell_price:
print("Selling Order for BTC is automated as {cost:,} dipped below buying price limit of {sell_price}")
auth_client.sell(size=sell_amount, order_type="market", product_id="BTC-USD"))
else:
print(f"null..... as price is {cost:,}!!")
time.sleep(5)
Conclusion
Apart from using the buy low and sell high strategy, you can also code other trading strategies you want these bots to follow. Remember that the strategy in this article is not recommended for trading purposes; you have to develop your own strategy.