Telegram remains one of the best messaging and content sharing applications. It is one of the best messaging apps you can use majorly for business messaging campaigns.
You can send messages, photos, videos and files of any type (doc, zip, mp3, etc), create groups for up to 200,000 people or channels for broadcasting to unlimited audiences.
Telegram is available for free download from the Google Play store. It has no premium content or subscription costs and works across numerous devices. Telegram offers a lot of space for saving or downloading data and cloud storage.
Again, unlike other applications, it does not have a file-sharing capacity. Additionally, it has some built-in bots that regulate behavior or filter out user-sent spam.
So how do you begin writing a Python script to create your personalized Telegram bot? Read on to find out, but let’s understand what exactly a telegram bot is, how it works, and its benefits.
Table of Contents
- What are Telegram Bots?
- What are the Benefits of Telegram Bots?
- Getting Started With Building Your Telegram Bot in Python
- Conclusion
What are Telegram Bots?
Telegram bots are essentially software-controlled Telegram accounts with sort of AI characteristics. It is a unique kind of user that may perform a variety of tasks, including sending messages, setting reminders, playing music, taking orders, integrating with other services, and sending commands over the Internet.
Chatbots automate customer service via text or text-to-speech and conduct online chat chats. You can get updates about a specific service from these chatbots. Users are made aware of the services offered by the bots’ owner company.
Some of the top features Telegram bots provide are helpful to businesses. Chatbots can be used for social media platforms, arranging appointments, and providing reminders.
When you require information from virtually any area, a Telegram bot is a good way to get it quickly. Different bots perform different operations when triggered.
For example:
- @TriviaBot: Find out how much you know about trivia, or join groups to challenge others.
- @ImageBot: When you send this bot a phrase, it will respond with a corresponding image.
- @PollBot: To make auto polls, add this bot to group chats.
How Telegram Bots Works
A Telegram Bot acts like a typical chat buddy and completes predefined tasks autonomously or upon request. Sending the bot the command “/help,” for instance, will cause it to emit all of the commands it is capable of as text feedback in the chat, as shown below.
- /services
- /contact
- /about
- /reviews
Everyone seems to be utilizing this robot-like tool to advertise businesses, from e-commerce businesses to healthcare institutions. These highly developed bots are proficient at speaking and conversing with people in a polite and friendly manner.
What are the Benefits of Telegram Bots?
There are several benefits of Telegram Bots. Here are some of these benefits:
- By linking them to a customer relationship management (CRM), ticketing system, or chat platform, Telegram bots can also offer customer service or gather leads.
- It streamlines communication between a user and a machine and enables seamless task execution between humans and machines.
- It can assist with newsletter delivery to current clients, and portfolio presentation.
- Businesses can use it to automate some interactions or run marketing campaigns.
- It assists organizations in keeping users interested for a sufficient amount of time and accumulates important data.
- They assist you in promoting new products, sending proactive notifications, and hastening the decision-making process for purchases.
- Customers may reach chatbots 24/7, and they are quick to answer any questions they may have regarding your service. By doing this, you can be sure that your consumers can always find a solution to their issues, regardless of the time.
You must adhere to specific logic and have a basic understanding of a programming language like Python to build a chatbot for Telegram.
Getting Started With Building Your Telegram Bot in Python
Building a telegram chatbot in Python has gained enormous popularity in the IT and commercial worlds. On the other hand, the Python programming language offers several advantages.
It promotes a huge community, excellent Telegram APIs, and is readable and simple to write.
Python may be used to easily create a chatbot for Telegram that only needs a few quick steps to finish. Let’s examine the prerequisites for beginning the process.
- A fully functional Telegram account and an internet-connected computer, smartphone, or other devices
- You must have Python set up on your Windows or Mac computer.
Let’s begin construction now that everything is set up.
Steps to Building Your First Telegram Bot in Python
A. The first phase is to set up the Telegram bot on your device
- Start by launching the Telegram app and entering “BotFather” into the search field. You must get in touch with BotFather, which is what you use to establish a chatbot on Telegram.
- Next, you have to click the “BotFather” button and “Start.”
- Before choosing your unique bot name, enter “/newbot”.
- Enter a special username for your bot now. Keep in mind that the username must end with the keyword “bot.”(e.g ‘Nitrolex_bot’ or ‘Nitrolexbot’)
- You will receive a message containing a token to access the HTTP API after providing a distinct username. You will need your token when we begin working in the development environment, so keep it safe and secure.
An example showing the message containing your unique token.
"Done! Congratulations on your new bot. You will find it at t.me/Nitrolex_bot. You can now add a description, about section, and profile picture for your bot. See /help for a list of commands. By the way, when you've finished creating your bot, ping our Bot Support if you want a better username for it. Just make sure the bot is fully operational before you do this. Use this token to access the HTTP API: 5118217484:AABBHXbYJkbjMcowJDlpXwlSvJtmCXsw1v0 Keep your token secure and store it safely. It can be used by anyone to control your bot. For a description of the Bot API, see this page: https://core.telegram.org/bots/api."
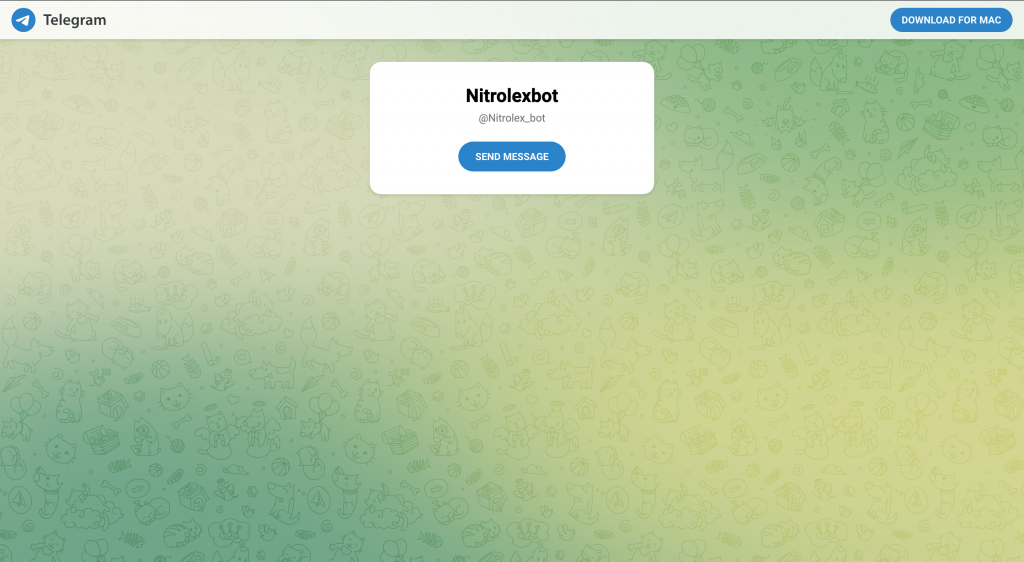
Once it has been set up, launch the virtual environment where you will be writing your Python code. By using a virtual environment, you can specify numerous locations on your computer, each with a unique collection of libraries and versions. You can use Google Colab or another Python environment in place of Visual Studio, which we’ll be using for this lesson.
B. The second phase is centered on installing the required modules and coding in the Python environment
- Ensure Python is installed before launching your IDE.
- Additionally, Python-Telegram-Bot must be installed as a module in the terminal or CMD. This module can be installed with pip and conda using the commands that are listed below.
- # installing via pip
- pip install python-telegram-bot
- # installing via conda
- conda install -c conda-forge python-telegram-bot
pip install python-telegram-bot
#------ or ------------#
conda install -c conda-forge python-telegram-bot
- If you are sure that the module is installed correctly, open two new files(name them Main and Reply) on your VSC. Below are samples of the codes you can include in these files.
Sample codes for Building Telegram Bots in Python
A. The “Main” file should amass the following Python codes:
from telegram import *
from telegram.ext import *
import Reply
# enter the token from your telegram bot here
updater = Updater('5118217484:AABBHXbYJkbjMcowJDlpXwlSvJtmCXsw1v0', use_context= True)
#this is the start up function, the first interaction with the bot
def start(update: Update, context: CallbackContext):
update.message.reply_text("hey, I am available to text and interact with you. Enter /help to see more options
")
def help(update: Update, context: (CallbackContext):
update.message.reply_text("""
Enter /services to learn about the services we offer.
Enter /contact to know other ways to reach out to us.
""")
def services(update: Update,context: CallbackContext):
update.message.reply_text("""
We offer writing services on the following Niche:
1. Computer programming
2. Cryptocurrencies
3. NFTs
4. Blockchain
5. Metaverse
6. Personal Finance as well
""")
def contact(update: Update,context: CallbackContext):
update.message.reply_text("contact us on tannerabraham365@gmail.com")
def messages(update: Update, context: CallbackContext):
text = str (update.message.text).lower()
response = Reply.sample_messages(text)
update.message.reply_text(response)
def error(update: Update, context):
update.message.reply_text(("something went wrong and I am not here to help you")
print(f"Update {update} caused error {context.error}")
updater.dispatcher.add_handler(CommandHandler('start', start))
updater.dispatcher.add_handler(CommandHandler('help', help))
updater.dispatcher.add_handler(CommandHandler('services', services))
updater.dispatcher.add_handler(CommandHandler('contact', contact))
updater.dispatcher.add_handler(MessageHandler(Filters.text, messages))
updater.dispatcher.add_error_handler(error))
updater.start_polling()
B. The “Reply ” file should have the following Python codes:
from datetime import datetime
def sample_messages(user_msg):
user_msg = str(user_msg).lower()
if user_msg in ('hello', 'hi'):
return 'hey, hi'
if user_msg in ('how are you'):
return "I am fine, how are you"
if user_msg in ('I am fine'):
return 'that\'s good'
if user_msg in ('time'):
now = datetime.now()
date_time = now.strftime("%d/%m/%y", "%H:%M:%S")
return str(date_time)
return "Sorry, I am having a hard time understanding this."
Using this construct, you can add more functionality for additional services based on your industry. You can do this on separate files, then import them into the main file before running the code.
Conclusion
With business’s explosive growth and assurances of privacy and security, Telegram has emerged as a crucial platform for businesses to utilize. Additionally, it is becoming a more vital business application for various business messaging campaigns thanks to the Telegram chatbot.
A Telegram chatbot differs from a human agent in that it sometimes fails to comprehend a question or has a small pool of potential responses. This gives it a “robotic” feature. AI-based solutions let you give your chatbot a personality and have it adjust to the situation.