Have you ever come across a hex encoded string and wondered how to convert it back to readable text?
Hex Encoding
Hex encoding is a popular way to represent binary data, but it can be challenging to interpret without the right tools. In this article, we will explore how to convert a hex encoded string to ASCII using Python.
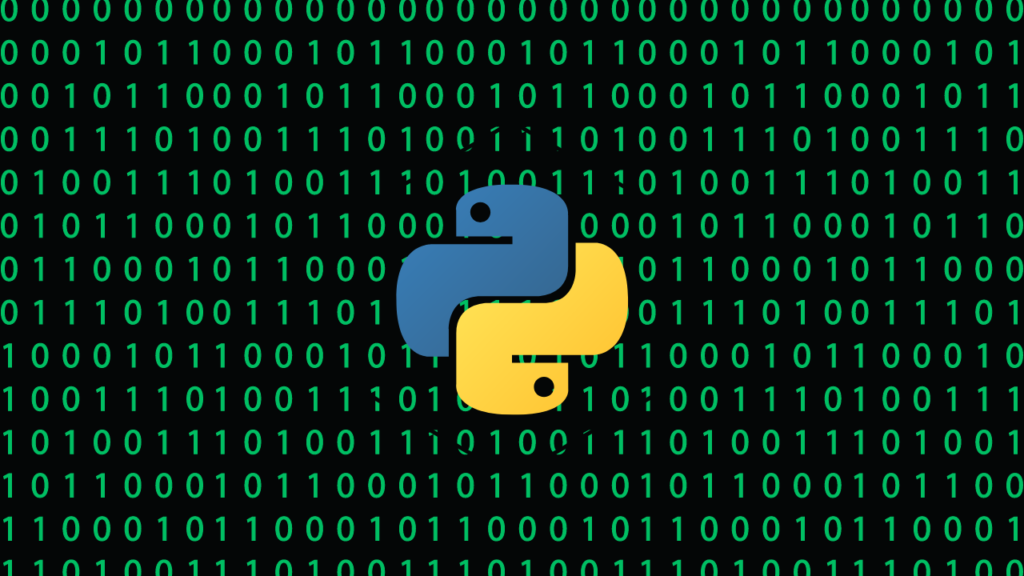
Table of Contents
- Hex Encoding
- Understanding Hexadecimal Encoding
- Converting Hex Encoded String to ASCII with Python
- Example: Converting a Hex Encoded String to ASCII
- Converting Hex Encoded String to ASCII: A Step-by-Step Guide
- Conclusion
- FAQs
What is a hex encoded string?
A hex encoded string is a representation of binary data using hexadecimal (base-16) digits.
It is often used in various contexts, such as encoding binary files or transmitting binary data over text-based protocols.
Each pair of hexadecimal digits represents a byte of data, allowing for a more compact representation compared to the corresponding ASCII or Unicode characters.
Why would you want to convert it to ASCII?
Converting a hex encoded string to ASCII is useful when you need to interpret the binary data as readable text.
For example, you might encounter hex encoded strings when working with cryptographic functions, analyzing network traffic, or parsing binary file formats.
By converting the hex encoded string to ASCII, you can easily examine and manipulate the data.
Understanding Hexadecimal Encoding
Before we dive into converting hex encoded strings to ASCII, let’s briefly review hexadecimal encoding.
Hexadecimal uses 16 distinct symbols: the digits 0-9 and the letters A-F. Each hexadecimal digit represents four bits of binary data.
For example, the digit “0” corresponds to the binary value 0000, “1” to 0001, “2” to 0010, and so on, up to “F” for 1111.
When converting a hex encoded string to ASCII, we need to reverse this process.
Each pair of hexadecimal digits represents one byte of binary data, which can be converted back to its corresponding ASCII character.
Converting Hex Encoded String to ASCII with Python
Python provides several ways to convert a hex encoded string to ASCII. One of the most straightforward methods is to use the binascii
module, which provides functions for binary data manipulation.
Method 1: Using binascii.unhexlify()
The binascii
module includes a function called unhexlify()
, which converts a hex encoded string to binary data.
We can then decode the binary data using the ASCII encoding to obtain the corresponding ASCII characters. Here’s an example:
import binascii
hex_string = "48656c6c6f20576f726c64" # Hex encoded string
binary_data = binascii.unhexlify(hex_string) # Convert to binary data
ascii_text = binary_data.decode("ascii") # Decode as ASCII
print(ascii_text) # Output: Hello World
Method 2: Using a loop and slicing
Another approach is to iterate over the hex encoded string, extract each pair of hexadecimal digits, convert them to their corresponding decimal values, and then convert the decimal values to ASCII characters. Here’s an example of this method:
hex_string = "48656c6c6f20576f726c64" # Hex encoded string
# Iterate over pairs of hexadecimal digits
ascii_text = ""
for i in range(0, len(hex_string), 2):
hex_pair = hex_string[i:i+2]
decimal_value = int(hex_pair, 16) # Convert hex to decimal
ascii_character = chr(decimal_value) # Convert decimal to ASCII
ascii_text += ascii_character
print(ascii_text) # Output: Hello World
Both methods achieve the same result, converting the hex encoded string “48656c6c6f20576f726c64” to the ASCII text “Hello World”.
Example: Converting a Hex Encoded String to ASCII
Let’s explore a more comprehensive example to solidify our understanding.
Suppose we have the hex encoded string “74657374696e6720696e20507974686f6e21”. Using the binascii.unhexlify()
method, we can convert it to ASCII:
import binascii
hex_string = "74657374696e6720696e20507974686f6e21" # Hex encoded string
binary_data = binascii.unhexlify(hex_string) # Convert to binary data
ascii_text = binary_data.decode("ascii") # Decode as ASCII
print(ascii_text) # Output: testing in Python!
In this example, the hex encoded string “74657374696e6720696e20507974686f6e21” is converted to the ASCII text “testing in Python!”.
Converting Hex Encoded String to ASCII: A Step-by-Step Guide
Now that we have explored the basics, let’s dive deeper into the process of converting a hex encoded string to ASCII using Python.
We will walk through a step-by-step guide that covers both the methods we discussed earlier.
Step 1: Import the necessary module
To get started, we need to import the binascii
module, which provides functions for binary data manipulation.
import binascii
Step 2: Obtain the hex encoded string
Next, we need a hex encoded string that we want to convert to ASCII. For example, let’s use the hex encoded string “4c6f72656d20697073756d2064616c6f7221”.
hex_string = "4c6f72656d20697073756d2064616c6f7221"
Step 3: Convert the hex encoded string to binary data
Now, we can use the binascii.unhexlify()
function to convert the hex encoded string to binary data.
binary_data = binascii.unhexlify(hex_string)
Step 4: Decode the binary data as ASCII
Once we have the binary data, we can decode it using the ASCII encoding to obtain the corresponding ASCII characters.
ascii_text = binary_data.decode("ascii")
Step 5: Print the converted ASCII text
Finally, we can print the converted ASCII text.
print(ascii_text)
Putting it all together, here’s the complete code:
import binascii
hex_string = "4c6f72656d20697073756d2064616c6f7221"
binary_data = binascii.unhexlify(hex_string)
ascii_text = binary_data.decode("ascii")
print(ascii_text)
Running this code will output the following:
Lorem ipsum dolor!
Conclusion
Converting a hex encoded string to ASCII using Python is a simple yet powerful technique for interpreting and manipulating binary data.
By understanding hexadecimal encoding and utilizing the appropriate functions, such as binascii.unhexlify()
and decoding with ASCII encoding, you can easily convert hex encoded strings to readable text.
In this article, we explored two methods for converting hex encoded strings to ASCII: using the binascii
module and manually iterating over the hex string.
We also provided a step-by-step guide to help you understand and implement the conversion process in your Python projects.
By mastering this skill, you can handle hex encoded data more effectively, enabling you to work with cryptographic functions, analyze network traffic, and parse binary file formats with ease.
FAQs
Q: Can I convert a hex encoded string to ASCII without using Python?
A: Yes, you can convert a hex encoded string to ASCII using other programming languages as well. The process involves converting the hex digits to their corresponding decimal values and then mapping those values to ASCII characters. Most programming languages provide built-in functions or methods for this conversion.
Q: What if the hex encoded string contains non-ASCII characters?
A: The conversion from hex encoded string to ASCII assumes that the original data consists of ASCII characters. If the hex encoded string contains non-ASCII characters or characters that do not have corresponding ASCII values, the conversion may result in unexpected or invalid output. It is important to ensure that the input hex encoded string represents valid ASCII characters before performing the conversion.
Q: Can I convert a hex encoded string to Unicode instead of ASCII?
A: Yes, you can convert a hex encoded string to Unicode using Python. Instead of decoding the binary data with the ASCII encoding, you can use other encodings such as UTF-8 or UTF-16. The choice of encoding depends on the specific requirements of your application and the character set you want to represent.
Q: Is there a limit to the length of the hex encoded string I can convert to ASCII?
A: In theory, there is no strict limit to the length of the hex encoded string you can convert to ASCII using Python. However, the practical limit depends on the available memory and system resources. If the hex encoded string is extremely large, it may consume excessive memory during the conversion process. It is advisable to consider memory constraints and optimize your code if dealing with very long hex encoded strings.
Q: Can I convert a hex encoded string with special characters to ASCII?
A: Yes, you can convert a hex encoded string with special characters to ASCII. The hex encoded string represents binary data, and as long as the binary data corresponds to valid ASCII characters, the conversion will work correctly. However, keep in mind that certain special characters may have specific representations or meanings in different contexts. It is essential to understand the context and intended use of the data to interpret the converted ASCII output accurately.