In today’s digital age, effective and engaging communication is crucial for businesses and individuals alike.
With the rise of chatbots and conversational AI, integrating popular messaging platforms with powerful language models has become a top priority.
In this article, we will explore how to seamlessly integrate Facebook Messenger with ChatGPT using Python, enabling you to create dynamic and interactive chatbot experiences.
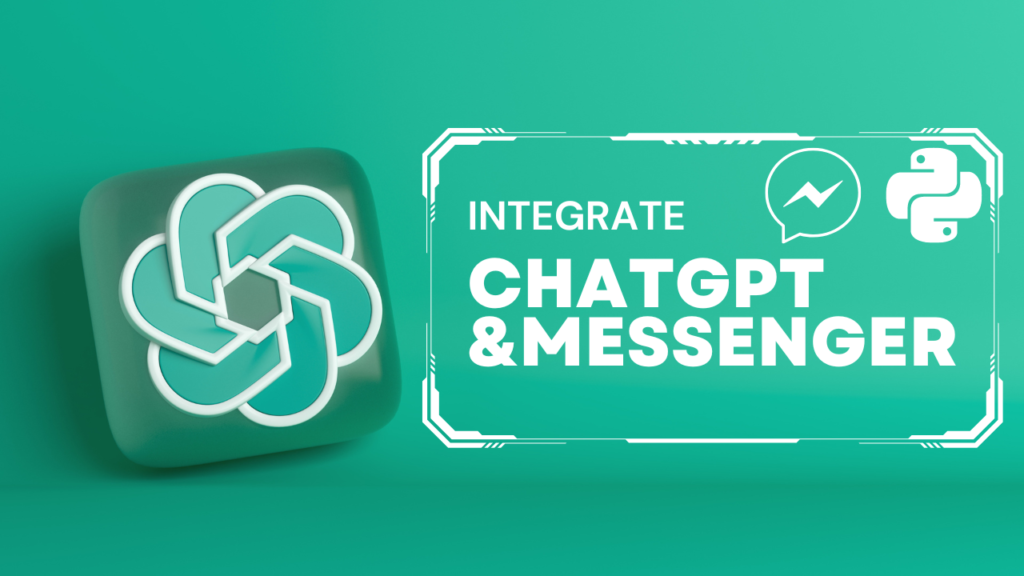
Table of Contents
- Understanding Facebook Messenger
- Introducing ChatGPT
- The Need for Integration
- Setting Up the Environment
- Authenticating with Facebook
- Designing the ChatGPT Backend
- Handling Incoming Messages
- Generating ChatGPT Responses
- Sending Responses to Messenger
- Testing and Debugging
- Deployment and Scaling
- Best Practices and Considerations
- Real-World Use Cases
- Conclusion
- FAQs
In recent years, Facebook Messenger has emerged as one of the leading messaging platforms, boasting billions of active users.
Its extensive features and user-friendly interface make it an ideal choice for businesses to connect with their customers. By integrating ChatGPT with Messenger, businesses can automate customer support, provide personalized recommendations, and enhance user experiences.
Understanding Facebook Messenger
Before diving into the integration process, let’s take a moment to understand the key features and benefits of Facebook Messenger.
As a versatile messaging platform, Messenger allows users to send text messages, make voice and video calls, share multimedia content, and even interact with businesses through chatbots.
By integrating ChatGPT with Messenger, businesses can leverage its features and provide automated and interactive conversational experiences.
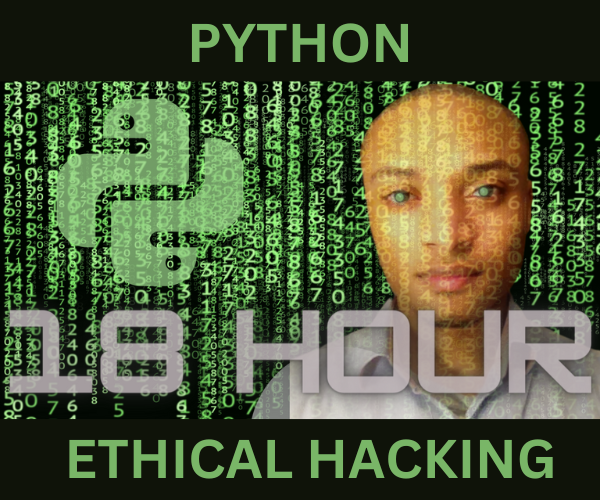
=> Join the Waitlist for Early Access.
Introducing ChatGPT
ChatGPT, developed by OpenAI, is a powerful language model that enables computers to engage in human-like conversations.
It has been trained on vast amounts of text data and can generate coherent and contextually relevant responses.
By integrating ChatGPT with Facebook Messenger, you can harness its natural language processing capabilities to create engaging and interactive chatbot experiences.
The Need for Integration
Integrating Facebook Messenger with ChatGPT brings numerous advantages to the table. Firstly, it allows businesses to automate their customer support, providing instant responses to frequently asked questions and common inquiries.
This reduces the workload on human support agents and enables faster resolution times.
Furthermore, integrating ChatGPT with Messenger enables businesses to provide personalized recommendations and assistance to users.
By understanding user queries and preferences, ChatGPT can offer tailored suggestions and solutions, enhancing the overall user experience.
Setting Up the Environment
To get started with integrating Facebook Messenger and ChatGPT, you need to set up the development environment. Begin by installing the necessary Python libraries:
pip install flask requests pymessenger
These libraries provide the tools and functionality required to interact with Facebook Messenger’s API.
Next, create a Facebook app and obtain the required API credentials. This involves registering your app on the Facebook Developer Portal, setting up the webhook URL, and configuring the necessary permissions.
Authenticating with Facebook
Authentication is a crucial step in integrating ChatGPT with Facebook Messenger.
To authenticate your app, you’ll need to generate an access token that grants the required permissions for interacting with the Messenger API.
This token ensures secure and authorized communication between your app and Messenger.
Here’s an example of how to generate the access token using Python:
import requests
def generate_access_token(app_id, app_secret):
url = f"https://graph.facebook.com/v13.0/oauth/access_token?client_id={app_id}&client_secret={app_secret}&grant_type=client_credentials"
response = requests.get(url)
access_token = response.json()["access_token"]
return access_token
# Replace 'APP_ID' and 'APP_SECRET' with your actual app credentials
access_token = generate_access_token('APP_ID', 'APP_SECRET')
Designing the ChatGPT Backend
To create a seamless integration, it’s important to design a robust and scalable backend for ChatGPT.
This backend will handle incoming messages from Messenger, process them using the ChatGPT model, and generate appropriate responses.
Here’s a basic example of how the backend can be structured using Flask:
from flask import Flask, request
from pymessenger import Bot
app = Flask(__name__)
bot = Bot('PAGE_ACCESS_TOKEN')
@app.route('/webhook', methods=['POST'])
def webhook():
data = request.get_json()
# Extract necessary information from the incoming message
sender_id = data['entry'][0]['messaging'][0]['sender']['id']
message_text = data['entry'][0]['messaging'][0]['message']['text']
# Process the message using ChatGPT model and generate a response
response = process_message(message_text)
# Send the response back to the user
bot.send_text_message(sender_id, response)
return 'Success'
def process_message(message):
# Use ChatGPT model to generate a response based on the message
# Replace this with your actual code for generating responses
return "This is the response generated by ChatGPT."
if __name__ == '__main__':
app.run(port=5000, debug=True)
Handling Incoming Messages
Once the backend is set up, you need to handle incoming messages from Facebook Messenger.
This involves configuring the webhook to receive messages and extracting the relevant information, such as the sender’s ID and the message content.
In the code snippet below, we handle incoming messages and extract the necessary information:
@app.route('/webhook', methods=['POST'])
def webhook():
data = request.get_json()
# Extract necessary information from the incoming message
sender_id = data['entry'][0]['messaging'][0]['sender']['id']
message_text = data['entry'][0]['messaging'][0]['message']['text']
# Process the message using ChatGPT model and generate a response
response = process_message(message_text)
# Send the response back to the user
bot.send_text_message(sender_id, response)
return 'Success'
Generating ChatGPT Responses
Once the incoming message is preprocessed, it’s time to generate a response using the ChatGPT model.
Pass the preprocessed message to the model and let it generate a coherent and contextually appropriate response. The generated response should consider the user’s query and provide valuable information or assistance.
In the code snippet below, we generate a simple response using the ChatGPT model:
def process_message(message):
# Use ChatGPT model to generate a response based on the message
# Replace this with your actual code for generating responses
return "This is the response generated by ChatGPT."
Sending Responses to Messenger
With the response generated, it’s time to send it back to Facebook Messenger. Use the Messenger API to craft a response message and send it to the user.
The response should be formatted in a way that appears natural and engaging, mimicking human conversation.
In the code snippet below, we send the response back to the user:
bot.send_text_message(sender_id, response)
Testing and Debugging
Testing and debugging are essential steps in ensuring the smooth functioning and reliability of the integration between Facebook Messenger and ChatGPT.
Thorough testing helps identify any issues or bugs, while debugging allows you to troubleshoot and fix them.
Here are some approaches and code examples to assist you in testing and debugging your integration:
Unit Testing
Unit testing involves testing individual components of your code to ensure they function as expected.
You can write unit tests for different parts of your integration, such as message processing, response generation, and API interactions.
Let’s take a look at an example of a unit test for the message processing function:
def test_process_message():
# Simulate an incoming message
message = "Hello, how can I help you?"
# Call the process_message function
response = process_message(message)
# Assert the expected response
assert response == "This is the response generated by ChatGPT."
In this example, we simulate an incoming message and verify that the response generated by the process_message
function matches the expected response.
Integration Testing
Integration testing involves testing the entire integration flow, from receiving a message to generating and sending a response.
This type of testing ensures that all the components work together seamlessly. Here’s an example of an integration test using the requests
library to simulate an incoming message and verify the response:
import requests
def test_integration():
# Simulate an incoming message
message = "Hello, how can I help you?"
sender_id = "USER_ID"
# Send a POST request to the webhook URL
response = requests.post('https://your-webhook-url.com/webhook', json={
"entry": [
{
"messaging": [
{
"sender": {
"id": sender_id
},
"message": {
"text": message
}
}
]
}
]
})
# Verify the response status code and content
assert response.status_code == 200
assert response.text == "Success"
In this example, we use the requests
library to send a POST request to the webhook URL, simulating an incoming message. We then verify that the response has a 200 status code and the expected content.
Logging and Error Handling
Logging and error handling are crucial for debugging and identifying issues in your integration.
You can use the logging
module to log important events, errors, or debug information during runtime.
Additionally, implementing error handling mechanisms allows you to catch and handle exceptions gracefully. Here’s an example of logging and error handling:
import logging
def process_message(message):
try:
# Process the message using ChatGPT model and generate a response
response = chatgpt.generate_response(message)
except Exception as e:
# Log the error
logging.error(f"An error occurred: {str(e)}")
# Return a default error message
response = "Apologies, but I'm experiencing some technical difficulties. Please try again later."
return response
In this example, we wrap the message processing logic in a try-except block. If an exception occurs, it is logged using the logging.error
function, and a default error message is returned.
By implementing logging and error handling, you can gather valuable information about any errors or unexpected behavior that may occur during the integration process.
Remember to thoroughly test and debug your integration, covering different scenarios and edge cases. This ensures that your integration functions reliably and provides an optimal user experience.
Deployment and Scaling
Once you have successfully developed and tested your integrated ChatGPT and Facebook Messenger application, it’s time to deploy it to a production environment and ensure scalability to handle increased user interactions.
Here, we’ll explore the deployment process and provide code examples for deploying and scaling your application using cloud platforms.
Deployment using Heroku
Heroku is a popular platform for deploying and hosting web applications. It offers a simple and straightforward deployment process.
To deploy your ChatGPT and Facebook Messenger integration using Heroku, follow these steps:
- Sign up for a free Heroku account at https://www.heroku.com/.
- Install the Heroku CLI (Command Line Interface) on your local machine.
- Open your command-line interface and navigate to your project directory.
- Create a new Heroku application by running the following command:
heroku create
- Commit your code changes to Git using the following commands:
git init
git add .
git commit -m "Initial commit"
- Deploy your application to Heroku by running the following command:
git push heroku master
- Once the deployment process is complete, Heroku will provide you with a URL for your application. You can use this URL to access your integrated ChatGPT and Facebook Messenger application.
Scaling using AWS Elastic Beanstalk
AWS Elastic Beanstalk is a fully managed service that makes it easy to deploy and scale applications. It provides automatic scaling capabilities and handles the underlying infrastructure for you.
To deploy and scale your ChatGPT and Facebook Messenger integration using AWS Elastic Beanstalk, follow these steps:
- Sign up for an AWS account at https://aws.amazon.com/.
- Install the AWS CLI (Command Line Interface) on your local machine.
- Open your command-line interface and navigate to your project directory.
- Initialize your project as an Elastic Beanstalk application by running the following command:
eb init -p python-3.7 my-application
- Create an environment and deploy your application to Elastic Beanstalk by running the following command:
eb create my-environment
- Once the deployment process is complete, Elastic Beanstalk will provide you with a URL for your application. You can use this URL to access your integrated ChatGPT and Facebook Messenger application.
- To scale your application, you can use the Elastic Beanstalk management console or the AWS CLI to adjust the instance capacity and enable auto-scaling based on your application’s needs.
By deploying your application to cloud platforms like Heroku or AWS Elastic Beanstalk, you can ensure scalability and reliability, allowing your integrated ChatGPT and Facebook Messenger application to handle increased user interactions without performance issues.
Remember to monitor your application’s performance and make necessary adjustments to ensure optimal scalability and user experience.
Best Practices and Considerations
When integrating Facebook Messenger with ChatGPT, it’s important to follow best practices and consider certain factors. Here are some key considerations:
1. Security and Privacy
Ensure that you handle user data and conversations securely, adhering to privacy regulations and industry standards. Implement measures such as data encryption and access controls to protect user information.
# Encrypt sensitive data
import hashlib
hashed_data = hashlib.sha256(data.encode()).hexdigest()
2. Model Updates and Fine-tuning
Regularly update and fine-tune your ChatGPT model to improve its performance and accuracy. OpenAI frequently releases new versions and improvements, so staying up to date ensures you provide the best chatbot experience to your users.
# Fine-tuning the model
from transformers import GPT2LMHeadModel, GPT2Tokenizer
model = GPT2LMHeadModel.from_pretrained('gpt2')
tokenizer = GPT2Tokenizer.from_pretrained('gpt2')
# Fine-tune the model with additional training data
model.train()
# Save the fine-tuned model for future use
model.save_pretrained('fine_tuned_model')
3. Ethical Implications
Consider the ethical implications of deploying a chatbot.
Ensure your bot behaves responsibly and avoids biases, offensive language, or harmful content. Implement moderation mechanisms and review user interactions to maintain a positive and inclusive user experience.
# Moderation and content filtering
def filter_content(response):
if contains_offensive_language(response):
response = replace_offensive_language(response)
if contains_sensitive_content(response):
response = filter_sensitive_content(response)
return response
4. Performance Optimization
Optimize the performance of your integration to ensure smooth and efficient user experiences. Implement techniques such as caching, request batching, or parallel processing to handle a high volume of user interactions.
# Caching responses
import redis
cache = redis.Redis(host='localhost', port=6379)
def get_cached_response(key):
if cache.exists(key):
return cache.get(key)
else:
response = generate_response()
cache.set(key, response)
return response
5. Error Handling and Logging
Implement robust error handling and logging mechanisms to track and troubleshoot any issues that may arise. Log relevant information, such as user requests, error messages, and system responses, for debugging and analysis.
# Error handling and logging
import logging
logging.basicConfig(filename='chatbot.log', level=logging.ERROR)
try:
# Code that may raise an exception
response = generate_response()
except Exception as e:
logging.error(f'Error occurred: {str(e)}')
response = 'Apologies, an error occurred. Please try again later.'
By incorporating these best practices into your integration, you can ensure the security, reliability, and ethical operation of your ChatGPT-powered Facebook Messenger chatbot.
Real-World Use Cases
Integrating Facebook Messenger with ChatGPT opens up a range of possibilities across various industries. Let’s explore some real-world use cases and see how Python code can be utilized to enhance these scenarios.
1. E-commerce Personalization
For e-commerce businesses, integrating ChatGPT with Messenger can enable personalized product recommendations based on user preferences and purchase history. Chatbots can help users discover relevant products and make informed purchase decisions.
def generate_product_recommendation(user_id):
# Retrieve user preferences and purchase history
user_preferences = get_user_preferences(user_id)
purchase_history = get_purchase_history(user_id)
# Use ChatGPT model to generate personalized product recommendations
recommendations = chatgpt_generate_recommendations(user_preferences, purchase_history)
return recommendations
2. Healthcare Assistance
In the healthcare sector, chatbots integrated with ChatGPT can provide initial diagnosis and symptom analysis, helping users make informed decisions about seeking medical attention. They can offer general health advice, suggest remedies, or guide users to appropriate healthcare services.
def perform_symptom_analysis(symptoms):
# Use ChatGPT model to analyze symptoms and provide initial diagnosis
diagnosis = chatgpt_analyze_symptoms(symptoms)
return diagnosis
def provide_health_advice(user_id):
# Retrieve user health information
health_profile = get_user_health_profile(user_id)
# Use ChatGPT model to generate personalized health advice
advice = chatgpt_generate_health_advice(health_profile)
return advice
3. Travel and Hospitality Support
Integrating ChatGPT with Messenger can streamline customer support processes for businesses in the travel and hospitality sectors. Users can inquire about bookings, get assistance with itinerary planning, and receive real-time updates, enhancing their overall customer experience.
def handle_booking_inquiry(inquiry):
# Use ChatGPT model to process booking inquiries and provide relevant information
response = chatgpt_process_booking_inquiry(inquiry)
return response
def assist_with_itinerary_planning(user_id):
# Retrieve user preferences and travel details
user_preferences = get_user_preferences(user_id)
travel_details = get_travel_details(user_id)
# Use ChatGPT model to generate personalized itinerary suggestions
itinerary = chatgpt_generate_itinerary(user_preferences, travel_details)
return itinerary
By incorporating the above code examples into your integration, you can enhance user experiences and automate various tasks using ChatGPT’s language capabilities.
Remember to customize the code according to your specific requirements, ensuring that it aligns with your business logic and data models. Additionally, consider integrating with relevant APIs or databases to fetch and process data for generating personalized responses.
The combination of Facebook Messenger, ChatGPT, and Python empowers businesses to deliver exceptional conversational experiences in various domains.
Conclusion
Integrating Facebook Messenger with ChatGPT using Python unlocks the potential to create dynamic and engaging chatbot experiences.
By leveraging ChatGPT’s language capabilities and the extensive user base of Messenger, businesses can automate customer support, provide personalized recommendations, and enhance user interactions.
Remember to follow the outlined steps for setting up the environment, authenticating with Facebook, designing the backend, and handling incoming messages.
Test and debug thoroughly to ensure the integration functions seamlessly. Lastly, consider best practices, ethical considerations, and real-world use cases to maximize the benefits of integrating Facebook Messenger with ChatGPT.
FAQs
1. Can I integrate ChatGPT with platforms other than Facebook Messenger?
Yes, ChatGPT can be integrated with various messaging platforms and applications, depending on the available APIs and developer resources.
2. Is it necessary to have prior programming experience to integrate ChatGPT with Facebook Messenger?
While programming experience can be beneficial, following the provided guidelines and documentation can help you integrate ChatGPT with Facebook Messenger even as a beginner.
3. Can I customize the responses generated by ChatGPT?
Yes, you can customize the responses by fine-tuning the ChatGPT model or implementing additional logic in the backend to modify the generated output.
4. How can I handle complex user queries or requests using ChatGPT?
For complex queries, you can break them down into smaller parts and handle them individually. You can also combine ChatGPT with other APIs or services to enhance the functionality and response capabilities.
5. Is it possible to integrate ChatGPT with multiple messaging platforms simultaneously?
Yes, it is possible to integrate ChatGPT with multiple platforms by building separate integrations for each platform and handling their respective APIs.
Tanner Abraham
Data Scientist and Software Engineer with a focus on experimental projects in new budding technologies that incorporate machine learning and quantum computing into web applications.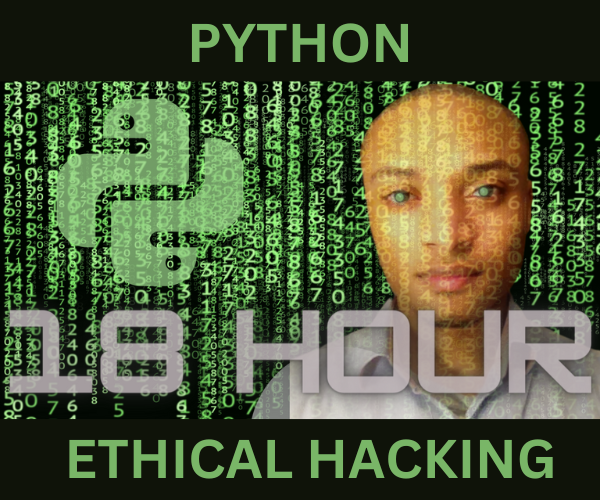
=> Join the Waitlist for Early Access.