Discord, a popular communication platform for gamers and online communities, allows users to create and manage their own servers.
These servers often require various features to enhance user experience and facilitate community management. One such feature is the addition of a Discord bot. In this article, we will explore how to create a ChatGPT Discord bot using Python, providing an interactive and engaging experience for users.
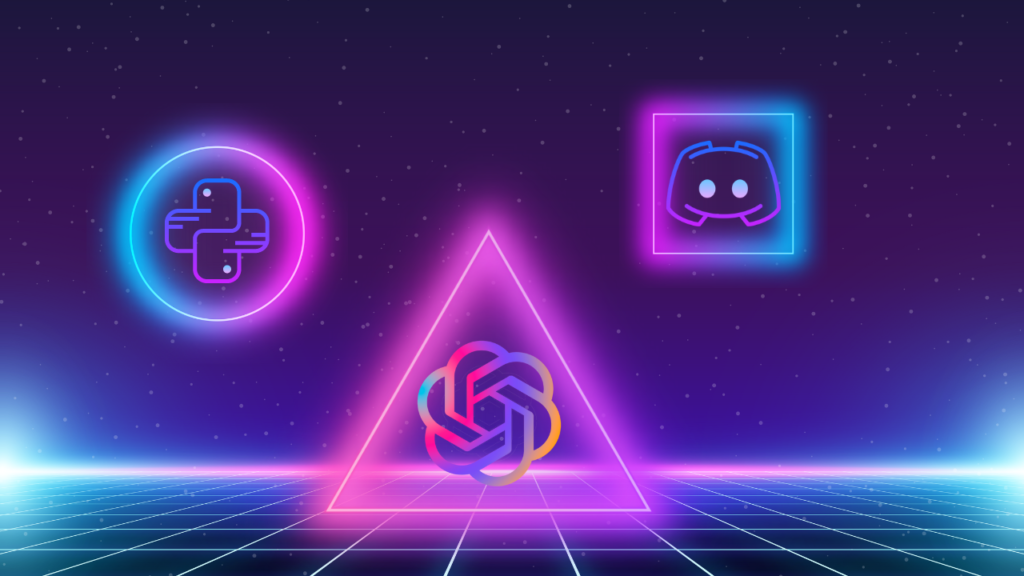
Table of Contents
- What is a Discord bot?
- Importance of Discord bots in community management
- Getting started with ChatGPT Discord bot
- Creating a Discord bot application
- Generating and storing the bot token
- Writing code for the ChatGPT Discord bot
- Handling user interactions and commands
- Customizing the bot’s responses
- Adding moderation features to the bot
- Deploying the ChatGPT Discord bot
- Testing and troubleshooting the bot
- Conclusion
- Frequently Asked Questions (FAQs)
Discord bots are automated programs that can perform a wide range of tasks within a Discord server. They can help with moderation, automation, entertainment, and much more. With the rise of artificial intelligence and natural language processing, ChatGPT has emerged as a powerful tool to create conversational bots. Integrating ChatGPT into a Discord bot allows users to interact with an AI-powered chatbot right within their server.
What is a Discord bot?
A Discord bot is essentially a user account created for a bot, rather than an actual human user. These bots can join servers and interact with users through text messages. They can be programmed to perform various actions based on predefined commands or triggered events. Discord bots have become an integral part of many communities, providing valuable functionality and entertainment.
Importance of Discord bots in community management
Discord bots play a crucial role in community management by automating repetitive tasks and adding valuable features. They can help in managing user roles, moderating content, organizing events, providing information, and even playing music. With a ChatGPT-powered Discord bot, users can have interactive conversations, ask questions, seek guidance, and engage in entertaining dialogues, creating a more immersive and dynamic community experience.
Getting started with ChatGPT Discord bot
To create a ChatGPT Discord bot, we will be using Python, a versatile programming language, and the Discord.py library, which provides a convenient interface for interacting with the Discord API. Let’s get started by setting up a Python environment.
Setting up a Python environment for the bot
- Install Python: Download and install the latest version of Python from the official Python website (https://www.python.org) based on your operating system.
- Create a virtual environment: Open your terminal or command prompt and navigate to your project directory. Run the following command to create a virtual environment:
python -m venv myenv
- Activate the virtual environment: Depending on your operating system, run the appropriate command to activate the virtual environment. For Windows:
myenv\Scripts\activate
For macOS and Linux:
source myenv/bin/activate
Installing the necessary packages
Once you have activated the virtual environment, we need to install the required packages. Run the following command to install Discord.py:
pip install discord.py
Additionally, we will need the OpenAI API package to integrate ChatGPT. Install it using the following command:
pip install openai
Creating a Discord bot application
To create a Discord bot, we need to set up a Discord bot application on the Discord Developer Portal. Follow these steps:
- Go to the Discord Developer Portal (https://discord.com/developers) and log in with your Discord account.
- Click on “New Application” and give your bot a name.
- Navigate to the “Bot” tab on the left-hand side menu and click on “Add Bot.”
- Customize your bot’s settings, such as its username and profile picture.
- Under the “Token” section, click on “Copy” to save your bot’s token. This token will be used to authenticate your bot when connecting to Discord servers.
Generating and storing the bot token
To securely store your bot token, we recommend using environment variables. Follow these steps:
- Create a file named “.env” in your project directory.
- Open the “.env” file and add the following line:
DISCORD_TOKEN=your_bot_token_here
Replace “your_bot_token_here” with the token you copied from the Discord Developer Portal.
- Save the file. Make sure to add “.env” to your project’s “.gitignore” file to prevent the token from being exposed in version control.
Writing code for the ChatGPT Discord bot
Now, let’s start writing the code for our ChatGPT Discord bot. Create a new Python file in your project directory and name it “bot.py”.
import discord
from discord.ext import commands
import os
import openai
# Load the bot token from the environment variable
TOKEN = os.getenv('DISCORD_TOKEN')
# Create a Discord client
bot = commands.Bot(command_prefix='!')
# Initialize the OpenAI API
openai.api_key = 'your_openai_api_key_here'
@bot.event
async def on_ready():
print(f'Logged in as {bot.user.name} ({bot.user.id})')
@bot.command()
async def chat(ctx, *args):
message = ' '.join(args)
response = openai.Completion.create(
engine='text-davinci-003',
prompt=message,
max_tokens=50,
temperature=0.7,
n=1,
stop=None,
timeout=15
)
await ctx.send(response.choices[0].text.strip())
bot.run(TOKEN)
Handling user interactions and commands
In the code above, we defined a simple command called “!chat”. When a user sends a message starting with “!chat”, the bot will use the OpenAI API to generate a response based on the user’s input.
To add more commands and customize the bot’s behavior, you can explore the Discord.py documentation (https://discordpy.readthedocs.io/en/stable/).
Customizing the bot’s responses
By modifying the parameters in the openai.Completion.create()
method, you can customize the bot’s responses. You can adjust the max_tokens
parameter to control the length of the response and experiment with different values for temperature
to vary the response’s creativity.
Remember to ensure that the bot’s responses comply with the Discord terms of service and community guidelines.
Adding moderation features to the bot
Apart from chat capabilities, you might want to add moderation features to your Discord bot. This can include tasks like banning users, deleting messages, or managing roles. Discord.py provides various functionalities to implement moderation features. You can explore the Discord.py documentation for more details on moderation features (https://discordpy.readthedocs.io/en/stable/api.html).
Deploying the ChatGPT Discord bot
To deploy the ChatGPT Discord bot, you have a few options depending on your requirements and preferences.
Self-hosting the bot
Self-hosting the bot allows you to have full control over its deployment. Here are the general steps to self-host your ChatGPT Discord bot:
- Set up a server or use an existing server where you can host your bot.
- Ensure that the server has the necessary dependencies, including Python and the required packages (Discord.py and OpenAI).
- Transfer your code and any additional files to the server.
- Run the bot by executing the
bot.py
file using the Python interpreter.
python bot.py
Make sure the bot is running and connected to the Discord server.
Using a cloud hosting platform
If you prefer not to self-host your bot or want to take advantage of cloud infrastructure, you can use a cloud hosting platform. Some popular choices include Heroku, AWS, Google Cloud, and Azure. The specific steps for deploying your bot may vary depending on the platform you choose.
- Create an account on your preferred cloud hosting platform and set up a new project or application.
- Follow the platform’s documentation to deploy your ChatGPT Discord bot. This typically involves configuring the necessary environment variables, specifying the runtime environment, and deploying your code.
- Once deployed, your bot should be up and running and ready to join your Discord server.
Testing and troubleshooting the bot
After deploying your ChatGPT Discord bot, it’s important to thoroughly test it and ensure it functions as intended. Test various commands, interactions, and scenarios to identify and address any issues. Here are some tips for testing and troubleshooting:
- Test the bot’s responses in different situations and ensure they align with your expectations. Adjust the OpenAI API parameters if needed.
- Use debugging tools and log messages to track the flow of your code and identify any errors or unexpected behavior.
- Gather feedback from users and iterate on your bot’s functionality based on their suggestions and needs.
Remember to keep your bot up to date with the latest versions of Discord.py and any other packages used to maintain compatibility and security.
Conclusion
Creating a ChatGPT Discord bot using Python and Discord.py opens up exciting possibilities for interactive and engaging experiences within Discord communities. By integrating ChatGPT, you can provide users with AI-powered conversations, assistance, and entertainment. Follow the steps outlined in this article to get started and customize your bot based on your specific requirements.
Building and deploying a ChatGPT Discord bot requires a combination of programming skills, creativity, and attention to detail. As you experiment and iterate on your bot, you’ll discover new ways to enhance user experiences and contribute to vibrant and dynamic Discord communities.
If you’re ready to create your own ChatGPT Discord bot, get started now and enjoy the benefits it brings to your community!
Frequently Asked Questions (FAQs)
1. Can I deploy multiple instances of the ChatGPT Discord bot?
Yes, you can deploy multiple instances of the ChatGPT Discord bot. Each instance will have its own unique bot token and can be hosted on different servers or cloud platforms.
2. How can I handle errors and exceptions in my Discord bot?
In Discord.py, you can use error handlers to handle specific exceptions that may occur during bot commands or events. This allows you to provide customized error messages and handle errors gracefully. Refer to the Discord.py documentation for more information on error handling.
3. Can I add additional features to my ChatGPT Discord bot?
Definitely! You can expand the functionality of your ChatGPT Discord bot by adding additional commands and features. Discord.py provides a wide range of APIs and events that you can utilize to customize and enhance your bot’s capabilities. Consider incorporating features like image manipulation, music playback, game integration, or even integration with external APIs to provide more interactive experiences for your Discord community.
4. How can I ensure the safety and moderation of my Discord server with the ChatGPT Discord bot?
To ensure the safety and moderation of your Discord server, it’s important to implement appropriate moderation features and guidelines. Discord.py offers various tools and functions for moderation, such as banning users, deleting messages, and managing roles. Additionally, you can set up profanity filters, implement user verification systems, and establish clear community guidelines to maintain a positive and inclusive environment.
5. Can I integrate other AI models or APIs with my ChatGPT Discord bot?
Yes, you can integrate other AI models or APIs with your ChatGPT Discord bot to enhance its functionality. For example, you can incorporate sentiment analysis models to analyze user sentiment, translation APIs to support multilingual interactions, or image recognition models for image-related commands. By leveraging various AI technologies, you can create a more versatile and dynamic bot that caters to the needs of your Discord community.
If you have any other questions or need further assistance with creating your ChatGPT Discord bot, feel free to reach out to the Discord.py community or consult the extensive documentation available.
Now that you have all the knowledge and tools necessary, it’s time to unleash your creativity and build your own ChatGPT Discord bot. Enjoy the process of crafting engaging interactions and providing valuable experiences for your Discord community. Happy bot-building!