Python has become a popular programming language among ethical hackers due to its simplicity, versatility, and an abundance of powerful libraries.
In this article, we will explore 10 essential Python libraries that every ethical hacker should be familiar with.
These libraries provide a wide range of functionalities, from network scanning and vulnerability assessment to cryptographic operations and exploit development.
Let’s dive in and discover the tools that will enhance your ethical hacking capabilities.
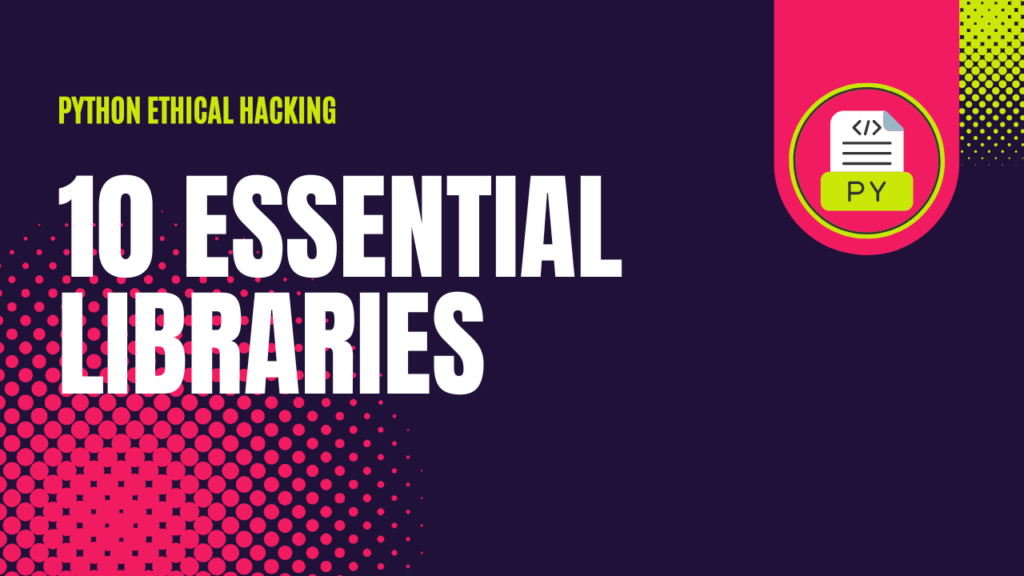
Table of Contents
- 1. Scapy – Crafting Network Packets with Python
- 2. Requests – Simplified HTTP Communication
- 3. Paramiko – SSHv2 Protocol Implementation
- 4. Beautiful Soup – HTML and XML Parsing
- 5. PyCrypto – Cryptographic Operations
- 6. Nmap – Network Discovery and Port Scanning
- 7. Impacket – Network Protocol Manipulation
- 8. PyCryptodome – Cryptographic Toolkit
- 9. Socket – Low-level Network Communication
- 10. Requests-HTML – Web Scraping and Parsing
- Conclusion
1. Scapy – Crafting Network Packets with Python
Scapy is a powerful library that allows for the manipulation of network packets at a low level.
It provides an extensive set of tools for packet creation, customization, sniffing, and sending.
With Scapy, ethical hackers can craft custom network packets to simulate various attacks, perform network reconnaissance, and analyze network traffic. Here’s a simple example that demonstrates how to send an ICMP packet:
from scapy.all import *
packet = IP(dst="192.168.0.1")/ICMP()
send(packet)
2. Requests – Simplified HTTP Communication
Requests is a popular library for making HTTP requests in Python.
It provides an easy-to-use interface for sending GET, POST, and other HTTP requests, handling cookies,
headers, and authentication.
Ethical hackers can leverage Requests to interact with web applications, perform web scraping, and exploit vulnerabilities. Here’s an example of making a GET request:
import requests
response = requests.get("https://example.com")
print(response.text)
3. Paramiko – SSHv2 Protocol Implementation
Paramiko is a Python library that implements the SSHv2 protocol, allowing secure and encrypted communication between machines.
Ethical hackers can use Paramiko for SSH authentication, remote command execution, file transfers, and building secure communication channels.
Here’s an example of connecting to an SSH server and executing a command:
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect("192.168.0.1", username="user", password="password")
stdin, stdout, stderr = ssh.exec_command("ls -l")
print(stdout.read().decode())
ssh.close()
4. Beautiful Soup – HTML and XML Parsing
Beautiful Soup is a Python library for parsing HTML and XML documents.
It provides a convenient and intuitive way to extract data from web pages, making it an essential tool for web scraping and data extraction in ethical hacking.
Here’s an example that demonstrates extracting all the links from a web page:
from bs4 import BeautifulSoup
import requests
response = requests.get("https://example.com")
soup = BeautifulSoup(response.text, "html.parser")
for link in soup.find_all("a"):
print(link.get("href"))
5. PyCrypto – Cryptographic Operations
PyCrypto is a comprehensive library for performing various cryptographic operations in
Python. It supports a wide range of encryption algorithms, digital signatures, hash functions, and secure random number generation.
Ethical hackers can utilize PyCrypto for tasks such as encrypting sensitive data, verifying digital signatures, and implementing secure communication protocols.
Here’s an example of encrypting and decrypting data using AES:
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
key = get_random_bytes(16)
cipher = AES.new(key, AES.MODE_EAX)
data = b"Hello, world!"
ciphertext, tag = cipher.encrypt_and_digest(data)
# ... Send ciphertext, tag, and nonce to the receiver
6. Nmap – Network Discovery and Port Scanning
Nmap is not a Python library per se, but it provides a powerful command-line tool that can be invoked from Python.
Nmap is widely used for network discovery, host scanning, and port scanning.
Ethical hackers can integrate Nmap into their Python scripts to automate network reconnaissance and identify open ports and services.
Here’s an example of using the subprocess
module to invoke Nmap and scan a target host:
import subprocess
target = "192.168.0.1"
command = ["nmap", "-p", "1-1000", target]
output = subprocess.check_output(command)
print(output.decode())
7. Impacket – Network Protocol Manipulation
Impacket is a collection of Python classes for working with network protocols.
It allows ethical hackers to manipulate network packets, perform network sniffing, and implement custom protocols.
Impacket provides support for common network protocols like SMB, SMB2, and LDAP, making it a valuable library for penetration testing and exploiting network vulnerabilities.
Here’s an example that demonstrates using Impacket to perform an SMB relay attack:
from impacket import smb, smbconnection
target = "192.168.0.1"
username = "user"
password = "password"
conn = smbconnection.SMBConnection(target, target, sess_port=445)
conn.login(username, password)
# ... Perform SMB relay attack or other operations
8. PyCryptodome – Cryptographic Toolkit
PyCryptodome is a fork of the PyCrypto library that offers a rich set of cryptographic functionalities. It provides support for symmetric and asymmetric encryption, digital signatures, hash functions, and more.
Ethical hackers can leverage PyCryptodome for tasks like encrypting and decrypting files, generating secure random numbers, and implementing secure protocols. Here’s an example of generating a SHA-256 hash:
from Cryptodome.Hash import SHA256
data = b"Hello, world!"
hash_object = SHA256.new(data)
hash_value = hash_object.hexdigest()
print(hash_value)
9. Socket – Low-level Network Communication
The socket
module is part of Python’s standard library and allows for low-level network communication.
Ethical hackers can use the socket
library to create custom network applications, interact with network services, and perform network-level operations.
Here’s an example of creating a TCP client:
import socket
target = "example.com"
port = 80
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((target, port))
# ... Send and receive data with the server
10. Requests-HTML – Web Scraping and Parsing
Requests-HTML is an extension of the Requests library that adds support for parsing HTML documents.
It simplifies web scraping tasks by providing a more intuitive and user-friendly API.
Ethical hackers can utilize Requests-HTML to extract data from web pages, scrape content, and automate web-based attacks.
Here’s an example of scraping headlines from a news website:
from requests_html import HTMLSession
session = HTMLSession()
response = session.get("https://example.com")
headlines = response.html.find(".headline")
for headline in headlines:
print(headline.text)
These 10 essential Python libraries empower ethical hackers to enhance their capabilities and automate various tasks in the field of cybersecurity.
Whether it’s crafting network packets, performing web scraping, or manipulating network protocols, these libraries provide the necessary tools to succeed.
Incorporate them into your ethical hacking toolkit and leverage their functionalities to bolster your expertise in the cybersecurity realm.
Remember to always use these tools responsibly and ethically, ensuring that you comply with legal and ethical guidelines in your endeavors.
As an aspiring ethical hacker, familiarizing yourself with these essential Python libraries will enable you to tackle a wide range of challenges in the field.
From network reconnaissance and vulnerability assessment to cryptographic operations and exploit development, these libraries provide a solid foundation for your ethical hacking journey.
Scapy offers unparalleled flexibility when it comes to network packet manipulation.
With its comprehensive set of tools, you can craft custom packets, analyze network traffic, and simulate various attacks.
Leveraging its capabilities, you can gain valuable insights into network vulnerabilities and devise effective defense strategies.
Requests simplifies HTTP communication, allowing you to interact with web applications, exploit vulnerabilities, and perform web scraping.
Its intuitive interface makes it easy to send requests, handle cookies, and authenticate, making it an invaluable tool for ethical hackers who rely on web-based attacks and information gathering.
Paramiko, an SSHv2 protocol implementation, empowers you to establish secure communication channels, execute remote commands, and transfer files.
Its robust capabilities are instrumental in securing remote connections and performing tasks such as privilege escalation and lateral movement.
Beautiful Soup excels at parsing HTML and XML documents, making it indispensable for web scraping and data extraction.
It’s intuitive API enables you to navigate through the structure of web pages, extract relevant information, and automate data gathering for further analysis and exploitation.
PyCrypto provides a comprehensive suite of cryptographic operations, including encryption, digital signatures, and secure random number generation.
By leveraging PyCrypto, you can secure sensitive data, verify the authenticity of digital assets, and implement secure communication protocols.
Nmap, although not a Python library, deserves a place in your ethical hacking toolkit.
This versatile network scanning and port scanning tool helps you discover hosts, identify open ports and services, and map out the network landscape.
Integrating Nmap into your Python scripts allows for automated reconnaissance and efficient vulnerability identification.
Impacket facilitates network protocol manipulation and network sniffing, providing you with the tools necessary for performing advanced attacks such as SMB relay attacks and protocol-level exploitation.
By leveraging Impacket, you can gain insights into network vulnerabilities, perform privilege escalation, and expand your arsenal of hacking techniques.
PyCryptodome, a fork of the PyCrypto library, extends its cryptographic functionalities.
With PyCryptodome, you can encrypt and decrypt files, generate secure random numbers, and implement advanced cryptographic algorithms to ensure the confidentiality and integrity of data.
The socket module, available in Python’s standard library, allows for low-level network communication.
With this library, you can create custom network applications, interact directly with network services, and perform network-level operations, providing you with fine-grained control over network connections.
Requests-HTML, an extension of the Requests library, simplifies web scraping tasks by providing an intuitive API for parsing HTML documents.
Leveraging Requests-HTML, you can extract data from web pages, automate web-based attacks, and gather critical intelligence for your ethical hacking endeavors.
By incorporating these 10 essential Python libraries into your ethical hacking arsenal, you equip yourself with the necessary tools to tackle various challenges in the cybersecurity landscape.
Whether it’s network exploration, web application testing, or cryptographic operations, these libraries provide a solid foundation for your journey towards becoming a proficient ethical hacker.
Embrace their functionalities, explore their capabilities, and let them empower you in your pursuit of securing digital systems and protecting against malicious threats.
Conclusion
In conclusion, these 10 essential Python libraries serve as the backbone of an ethical hacker’s toolkit.
They provide the necessary capabilities to perform a wide range of tasks, from network scanning and protocol manipulation to web scraping and cryptographic operations.
By leveraging the power of these libraries, you can enhance your skills, automate repetitive tasks, and stay ahead in the constantly evolving field of cybersecurity.
Remember, ethical hacking requires responsible and ethical use of these tools.
Always ensure that you have proper authorization and adhere to legal and ethical guidelines when conducting any hacking activities.
Use these libraries to protect and secure systems, identify vulnerabilities, and contribute to the overall improvement of cybersecurity practices.
As you embark on your journey from a software developer to a cybersecurity expert, make sure to continue expanding your knowledge and staying up-to-date with the latest trends and techniques in the field.
The cybersecurity landscape is ever-changing, and continuous learning and skill development are essential to remain effective and successful.
So, embrace these essential Python libraries, practice your skills, and continuously explore new possibilities.
With dedication, passion, and a solid foundation in these libraries, you can pave your way to becoming a proficient ethical hacker and contribute to a safer digital world.
Remember, ethical hacking is about using your skills and knowledge to make a positive impact.
Stay curious, stay ethical, and let these libraries be your allies on the exciting journey towards becoming a cybersecurity expert.
Happy hacking!
Note:
The use of these Python libraries should always be within the bounds of the law and ethical guidelines. It is crucial to obtain proper authorization and conduct activities responsibly.
This article is for educational purposes only and does not encourage any illegal or unethical activities.